Repo structure update for clarity
|
@ -1,545 +0,0 @@
|
|||
/*
|
||||
PS2Keyboard.cpp - PS2Keyboard library
|
||||
Copyright (c) 2007 Free Software Foundation. All right reserved.
|
||||
Written by Christian Weichel <info@32leaves.net>
|
||||
|
||||
** Mostly rewritten Paul Stoffregen <paul@pjrc.com> 2010, 2011
|
||||
** Modified for use beginning with Arduino 13 by L. Abraham Smith, <n3bah@microcompdesign.com> *
|
||||
** Modified for easy interrup pin assignement on method begin(datapin,irq_pin). Cuningan <cuninganreset@gmail.com> **
|
||||
|
||||
for more information you can read the original wiki in arduino.cc
|
||||
at http://www.arduino.cc/playground/Main/PS2Keyboard
|
||||
or http://www.pjrc.com/teensy/td_libs_PS2Keyboard.html
|
||||
|
||||
Version 2.4 (March 2013)
|
||||
- Support Teensy 3.0, Arduino Due, Arduino Leonardo & other boards
|
||||
- French keyboard layout, David Chochoi, tchoyyfr at yahoo dot fr
|
||||
|
||||
Version 2.3 (October 2011)
|
||||
- Minor bugs fixed
|
||||
|
||||
Version 2.2 (August 2011)
|
||||
- Support non-US keyboards - thanks to Rainer Bruch for a German keyboard :)
|
||||
|
||||
Version 2.1 (May 2011)
|
||||
- timeout to recover from misaligned input
|
||||
- compatibility with Arduino "new-extension" branch
|
||||
- TODO: send function, proposed by Scott Penrose, scooterda at me dot com
|
||||
|
||||
Version 2.0 (June 2010)
|
||||
- Buffering added, many scan codes can be captured without data loss
|
||||
if your sketch is busy doing other work
|
||||
- Shift keys supported, completely rewritten scan code to ascii
|
||||
- Slow linear search replaced with fast indexed table lookups
|
||||
- Support for Teensy, Arduino Mega, and Sanguino added
|
||||
|
||||
This library is free software; you can redistribute it and/or
|
||||
modify it under the terms of the GNU Lesser General Public
|
||||
License as published by the Free Software Foundation; either
|
||||
version 2.1 of the License, or (at your option) any later version.
|
||||
|
||||
This library is distributed in the hope that it will be useful,
|
||||
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
||||
Lesser General Public License for more details.
|
||||
|
||||
You should have received a copy of the GNU Lesser General Public
|
||||
License along with this library; if not, write to the Free Software
|
||||
Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
|
||||
*/
|
||||
|
||||
#include "PS2Keyboard.h"
|
||||
|
||||
#define BUFFER_SIZE 45
|
||||
static volatile uint8_t buffer[BUFFER_SIZE];
|
||||
static volatile uint8_t head, tail;
|
||||
static uint8_t DataPin;
|
||||
static uint8_t CharBuffer=0;
|
||||
static uint8_t UTF8next=0;
|
||||
static const PS2Keymap_t *keymap=NULL;
|
||||
|
||||
// The ISR for the external interrupt
|
||||
void ps2interrupt(void)
|
||||
{
|
||||
static uint8_t bitcount=0;
|
||||
static uint8_t incoming=0;
|
||||
static uint32_t prev_ms=0;
|
||||
uint32_t now_ms;
|
||||
uint8_t n, val;
|
||||
|
||||
val = digitalRead(DataPin);
|
||||
now_ms = millis();
|
||||
if (now_ms - prev_ms > 250) {
|
||||
bitcount = 0;
|
||||
incoming = 0;
|
||||
}
|
||||
prev_ms = now_ms;
|
||||
n = bitcount - 1;
|
||||
if (n <= 7) {
|
||||
incoming |= (val << n);
|
||||
}
|
||||
bitcount++;
|
||||
if (bitcount == 11) {
|
||||
uint8_t i = head + 1;
|
||||
if (i >= BUFFER_SIZE) i = 0;
|
||||
if (i != tail) {
|
||||
buffer[i] = incoming;
|
||||
head = i;
|
||||
}
|
||||
bitcount = 0;
|
||||
incoming = 0;
|
||||
}
|
||||
}
|
||||
|
||||
static inline uint8_t get_scan_code(void)
|
||||
{
|
||||
uint8_t c, i;
|
||||
|
||||
i = tail;
|
||||
if (i == head) return 0;
|
||||
i++;
|
||||
if (i >= BUFFER_SIZE) i = 0;
|
||||
c = buffer[i];
|
||||
tail = i;
|
||||
return c;
|
||||
}
|
||||
|
||||
// http://www.quadibloc.com/comp/scan.htm
|
||||
// http://www.computer-engineering.org/ps2keyboard/scancodes2.html
|
||||
|
||||
// These arrays provide a simple key map, to turn scan codes into ISO8859
|
||||
// output. If a non-US keyboard is used, these may need to be modified
|
||||
// for the desired output.
|
||||
//
|
||||
|
||||
const PROGMEM PS2Keymap_t PS2Keymap_US = {
|
||||
// without shift
|
||||
{0, PS2_F9, 0, PS2_F5, PS2_F3, PS2_F1, PS2_F2, PS2_F12,
|
||||
0, PS2_F10, PS2_F8, PS2_F6, PS2_F4, PS2_TAB, '`', 0,
|
||||
0, 0 /*Lalt*/, 0 /*Lshift*/, 0, 0 /*Lctrl*/, 'q', '1', 0,
|
||||
0, 0, 'z', 's', 'a', 'w', '2', 0,
|
||||
0, 'c', 'x', 'd', 'e', '4', '3', 0,
|
||||
0, ' ', 'v', 'f', 't', 'r', '5', 0,
|
||||
0, 'n', 'b', 'h', 'g', 'y', '6', 0,
|
||||
0, 0, 'm', 'j', 'u', '7', '8', 0,
|
||||
0, ',', 'k', 'i', 'o', '0', '9', 0,
|
||||
0, '.', '/', 'l', ';', 'p', '-', 0,
|
||||
0, 0, '\'', 0, '[', '=', 0, 0,
|
||||
0 /*CapsLock*/, 0 /*Rshift*/, PS2_ENTER /*Enter*/, ']', 0, '\\', 0, 0,
|
||||
0, 0, 0, 0, 0, 0, PS2_BACKSPACE, 0,
|
||||
0, '1', 0, '4', '7', 0, 0, 0,
|
||||
'0', '.', '2', '5', '6', '8', PS2_ESC, 0 /*NumLock*/,
|
||||
PS2_F11, '+', '3', '-', '*', '9', PS2_SCROLL, 0,
|
||||
0, 0, 0, PS2_F7 },
|
||||
// with shift
|
||||
{0, PS2_F9, 0, PS2_F5, PS2_F3, PS2_F1, PS2_F2, PS2_F12,
|
||||
0, PS2_F10, PS2_F8, PS2_F6, PS2_F4, PS2_TAB, '~', 0,
|
||||
0, 0 /*Lalt*/, 0 /*Lshift*/, 0, 0 /*Lctrl*/, 'Q', '!', 0,
|
||||
0, 0, 'Z', 'S', 'A', 'W', '@', 0,
|
||||
0, 'C', 'X', 'D', 'E', '$', '#', 0,
|
||||
0, ' ', 'V', 'F', 'T', 'R', '%', 0,
|
||||
0, 'N', 'B', 'H', 'G', 'Y', '^', 0,
|
||||
0, 0, 'M', 'J', 'U', '&', '*', 0,
|
||||
0, '<', 'K', 'I', 'O', ')', '(', 0,
|
||||
0, '>', '?', 'L', ':', 'P', '_', 0,
|
||||
0, 0, '"', 0, '{', '+', 0, 0,
|
||||
0 /*CapsLock*/, 0 /*Rshift*/, PS2_ENTER /*Enter*/, '}', 0, '|', 0, 0,
|
||||
0, 0, 0, 0, 0, 0, PS2_BACKSPACE, 0,
|
||||
0, '1', 0, '4', '7', 0, 0, 0,
|
||||
'0', '.', '2', '5', '6', '8', PS2_ESC, 0 /*NumLock*/,
|
||||
PS2_F11, '+', '3', '-', '*', '9', PS2_SCROLL, 0,
|
||||
0, 0, 0, PS2_F7 },
|
||||
0
|
||||
};
|
||||
|
||||
|
||||
const PROGMEM PS2Keymap_t PS2Keymap_German = {
|
||||
// without shift
|
||||
{0, PS2_F9, 0, PS2_F5, PS2_F3, PS2_F1, PS2_F2, PS2_F12,
|
||||
0, PS2_F10, PS2_F8, PS2_F6, PS2_F4, PS2_TAB, '^', 0,
|
||||
0, 0 /*Lalt*/, 0 /*Lshift*/, 0, 0 /*Lctrl*/, 'q', '1', 0,
|
||||
0, 0, 'y', 's', 'a', 'w', '2', 0,
|
||||
0, 'c', 'x', 'd', 'e', '4', '3', 0,
|
||||
0, ' ', 'v', 'f', 't', 'r', '5', 0,
|
||||
0, 'n', 'b', 'h', 'g', 'z', '6', 0,
|
||||
0, 0, 'm', 'j', 'u', '7', '8', 0,
|
||||
0, ',', 'k', 'i', 'o', '0', '9', 0,
|
||||
0, '.', '-', 'l', PS2_o_DIAERESIS, 'p', PS2_SHARP_S, 0,
|
||||
0, 0, PS2_a_DIAERESIS, 0, PS2_u_DIAERESIS, '\'', 0, 0,
|
||||
0 /*CapsLock*/, 0 /*Rshift*/, PS2_ENTER /*Enter*/, '+', 0, '#', 0, 0,
|
||||
0, '<', 0, 0, 0, 0, PS2_BACKSPACE, 0,
|
||||
0, '1', 0, '4', '7', 0, 0, 0,
|
||||
'0', '.', '2', '5', '6', '8', PS2_ESC, 0 /*NumLock*/,
|
||||
PS2_F11, '+', '3', '-', '*', '9', PS2_SCROLL, 0,
|
||||
0, 0, 0, PS2_F7 },
|
||||
// with shift
|
||||
{0, PS2_F9, 0, PS2_F5, PS2_F3, PS2_F1, PS2_F2, PS2_F12,
|
||||
0, PS2_F10, PS2_F8, PS2_F6, PS2_F4, PS2_TAB, PS2_DEGREE_SIGN, 0,
|
||||
0, 0 /*Lalt*/, 0 /*Lshift*/, 0, 0 /*Lctrl*/, 'Q', '!', 0,
|
||||
0, 0, 'Y', 'S', 'A', 'W', '"', 0,
|
||||
0, 'C', 'X', 'D', 'E', '$', PS2_SECTION_SIGN, 0,
|
||||
0, ' ', 'V', 'F', 'T', 'R', '%', 0,
|
||||
0, 'N', 'B', 'H', 'G', 'Z', '&', 0,
|
||||
0, 0, 'M', 'J', 'U', '/', '(', 0,
|
||||
0, ';', 'K', 'I', 'O', '=', ')', 0,
|
||||
0, ':', '_', 'L', PS2_O_DIAERESIS, 'P', '?', 0,
|
||||
0, 0, PS2_A_DIAERESIS, 0, PS2_U_DIAERESIS, '`', 0, 0,
|
||||
0 /*CapsLock*/, 0 /*Rshift*/, PS2_ENTER /*Enter*/, '*', 0, '\'', 0, 0,
|
||||
0, '>', 0, 0, 0, 0, PS2_BACKSPACE, 0,
|
||||
0, '1', 0, '4', '7', 0, 0, 0,
|
||||
'0', '.', '2', '5', '6', '8', PS2_ESC, 0 /*NumLock*/,
|
||||
PS2_F11, '+', '3', '-', '*', '9', PS2_SCROLL, 0,
|
||||
0, 0, 0, PS2_F7 },
|
||||
1,
|
||||
// with altgr
|
||||
{0, PS2_F9, 0, PS2_F5, PS2_F3, PS2_F1, PS2_F2, PS2_F12,
|
||||
0, PS2_F10, PS2_F8, PS2_F6, PS2_F4, PS2_TAB, 0, 0,
|
||||
0, 0 /*Lalt*/, 0 /*Lshift*/, 0, 0 /*Lctrl*/, '@', 0, 0,
|
||||
0, 0, 0, 0, 0, 0, PS2_SUPERSCRIPT_TWO, 0,
|
||||
0, 0, 0, 0, PS2_CURRENCY_SIGN, 0, PS2_SUPERSCRIPT_THREE, 0,
|
||||
0, 0, 0, 0, 0, 0, 0, 0,
|
||||
0, 0, 0, 0, 0, 0, 0, 0,
|
||||
0, 0, PS2_MICRO_SIGN, 0, 0, '{', '[', 0,
|
||||
0, 0, 0, 0, 0, '}', ']', 0,
|
||||
0, 0, 0, 0, 0, 0, '\\', 0,
|
||||
0, 0, 0, 0, 0, 0, 0, 0,
|
||||
0 /*CapsLock*/, 0 /*Rshift*/, PS2_ENTER /*Enter*/, '~', 0, '#', 0, 0,
|
||||
0, '|', 0, 0, 0, 0, PS2_BACKSPACE, 0,
|
||||
0, '1', 0, '4', '7', 0, 0, 0,
|
||||
'0', '.', '2', '5', '6', '8', PS2_ESC, 0 /*NumLock*/,
|
||||
PS2_F11, '+', '3', '-', '*', '9', PS2_SCROLL, 0,
|
||||
0, 0, 0, PS2_F7 }
|
||||
};
|
||||
|
||||
|
||||
const PROGMEM PS2Keymap_t PS2Keymap_French = {
|
||||
// without shift
|
||||
{0, PS2_F9, 0, PS2_F5, PS2_F3, PS2_F1, PS2_F2, PS2_F12,
|
||||
0, PS2_F10, PS2_F8, PS2_F6, PS2_F4, PS2_TAB, PS2_SUPERSCRIPT_TWO, 0,
|
||||
0, 0 /*Lalt*/, 0 /*Lshift*/, 0, 0 /*Lctrl*/, 'a', '&', 0,
|
||||
0, 0, 'w', 's', 'q', 'z', PS2_e_ACUTE, 0,
|
||||
0, 'c', 'x', 'd', 'e', '\'', '"', 0,
|
||||
0, ' ', 'v', 'f', 't', 'r', '(', 0,
|
||||
0, 'n', 'b', 'h', 'g', 'y', '-', 0,
|
||||
0, 0, ',', 'j', 'u', PS2_e_GRAVE, '_', 0,
|
||||
0, ';', 'k', 'i', 'o', PS2_a_GRAVE, PS2_c_CEDILLA, 0,
|
||||
0, ':', '!', 'l', 'm', 'p', ')', 0,
|
||||
0, 0, PS2_u_GRAVE, 0, '^', '=', 0, 0,
|
||||
0 /*CapsLock*/, 0 /*Rshift*/, PS2_ENTER /*Enter*/, '$', 0, '*', 0, 0,
|
||||
0, '<', 0, 0, 0, 0, PS2_BACKSPACE, 0,
|
||||
0, '1', 0, '4', '7', 0, 0, 0,
|
||||
'0', '.', '2', '5', '6', '8', PS2_ESC, 0 /*NumLock*/,
|
||||
PS2_F11, '+', '3', '-', '*', '9', PS2_SCROLL, 0,
|
||||
0, 0, 0, PS2_F7 },
|
||||
// with shift
|
||||
{0, PS2_F9, 0, PS2_F5, PS2_F3, PS2_F1, PS2_F2, PS2_F12,
|
||||
0, PS2_F10, PS2_F8, PS2_F6, PS2_F4, PS2_TAB, 0, 0,
|
||||
0, 0 /*Lalt*/, 0 /*Lshift*/, 0, 0 /*Lctrl*/, 'A', '1', 0,
|
||||
0, 0, 'W', 'S', 'Q', 'Z', '2', 0,
|
||||
0, 'C', 'X', 'D', 'E', '4', '3', 0,
|
||||
0, ' ', 'V', 'F', 'T', 'R', '5', 0,
|
||||
0, 'N', 'B', 'H', 'G', 'Y', '6', 0,
|
||||
0, 0, '?', 'J', 'U', '7', '8', 0,
|
||||
0, '.', 'K', 'I', 'O', '0', '9', 0,
|
||||
0, '/', PS2_SECTION_SIGN, 'L', 'M', 'P', PS2_DEGREE_SIGN, 0,
|
||||
0, 0, '%', 0, PS2_DIAERESIS, '+', 0, 0,
|
||||
0 /*CapsLock*/, 0 /*Rshift*/, PS2_ENTER /*Enter*/, PS2_POUND_SIGN, 0, PS2_MICRO_SIGN, 0, 0,
|
||||
0, '>', 0, 0, 0, 0, PS2_BACKSPACE, 0,
|
||||
0, '1', 0, '4', '7', 0, 0, 0,
|
||||
'0', '.', '2', '5', '6', '8', PS2_ESC, 0 /*NumLock*/,
|
||||
PS2_F11, '+', '3', '-', '*', '9', PS2_SCROLL, 0,
|
||||
0, 0, 0, PS2_F7 },
|
||||
1,
|
||||
// with altgr
|
||||
{0, PS2_F9, 0, PS2_F5, PS2_F3, PS2_F1, PS2_F2, PS2_F12,
|
||||
0, PS2_F10, PS2_F8, PS2_F6, PS2_F4, PS2_TAB, 0, 0,
|
||||
0, 0 /*Lalt*/, 0 /*Lshift*/, 0, 0 /*Lctrl*/, '@', 0, 0,
|
||||
0, 0, 0, 0, 0, 0, '~', 0,
|
||||
0, 0, 0, 0, 0 /*PS2_EURO_SIGN*/, '{', '#', 0,
|
||||
0, 0, 0, 0, 0, 0, '[', 0,
|
||||
0, 0, 0, 0, 0, 0, '|', 0,
|
||||
0, 0, 0, 0, 0, '`', '\\', 0,
|
||||
0, 0, 0, 0, 0, '@', '^', 0,
|
||||
0, 0, 0, 0, 0, 0, ']', 0,
|
||||
0, 0, 0, 0, 0, 0, '}', 0,
|
||||
0 /*CapsLock*/, 0 /*Rshift*/, PS2_ENTER /*Enter*/, '¤', 0, '#', 0, 0,
|
||||
0, '|', 0, 0, 0, 0, PS2_BACKSPACE, 0,
|
||||
0, '1', 0, '4', '7', 0, 0, 0,
|
||||
'0', '.', '2', '5', '6', '8', PS2_ESC, 0 /*NumLock*/,
|
||||
PS2_F11, '+', '3', '-', '*', '9', PS2_SCROLL, 0,
|
||||
0, 0, 0, PS2_F7 }
|
||||
};
|
||||
|
||||
|
||||
#define BREAK 0x01
|
||||
#define MODIFIER 0x02
|
||||
#define SHIFT_L 0x04
|
||||
#define SHIFT_R 0x08
|
||||
#define ALTGR 0x10
|
||||
|
||||
static char get_iso8859_code(void)
|
||||
{
|
||||
static uint8_t state=0;
|
||||
uint8_t s;
|
||||
char c;
|
||||
|
||||
while (1) {
|
||||
s = get_scan_code();
|
||||
if (!s) return 0;
|
||||
if (s == 0xF0) {
|
||||
state |= BREAK;
|
||||
} else if (s == 0xE0) {
|
||||
state |= MODIFIER;
|
||||
} else {
|
||||
if (state & BREAK) {
|
||||
if (s == 0x12) {
|
||||
state &= ~SHIFT_L;
|
||||
} else if (s == 0x59) {
|
||||
state &= ~SHIFT_R;
|
||||
} else if (s == 0x11 && (state & MODIFIER)) {
|
||||
state &= ~ALTGR;
|
||||
}
|
||||
// CTRL, ALT & WIN keys could be added
|
||||
// but is that really worth the overhead?
|
||||
state &= ~(BREAK | MODIFIER);
|
||||
continue;
|
||||
}
|
||||
if (s == 0x12) {
|
||||
state |= SHIFT_L;
|
||||
continue;
|
||||
} else if (s == 0x59) {
|
||||
state |= SHIFT_R;
|
||||
continue;
|
||||
} else if (s == 0x11 && (state & MODIFIER)) {
|
||||
state |= ALTGR;
|
||||
}
|
||||
c = 0;
|
||||
if (state & MODIFIER) {
|
||||
switch (s) {
|
||||
case 0x70: c = PS2_INSERT; break;
|
||||
case 0x6C: c = PS2_HOME; break;
|
||||
case 0x7D: c = PS2_PAGEUP; break;
|
||||
case 0x71: c = PS2_DELETE; break;
|
||||
case 0x69: c = PS2_END; break;
|
||||
case 0x7A: c = PS2_PAGEDOWN; break;
|
||||
case 0x75: c = PS2_UPARROW; break;
|
||||
case 0x6B: c = PS2_LEFTARROW; break;
|
||||
case 0x72: c = PS2_DOWNARROW; break;
|
||||
case 0x74: c = PS2_RIGHTARROW; break;
|
||||
case 0x4A: c = '/'; break;
|
||||
case 0x5A: c = PS2_ENTER; break;
|
||||
default: break;
|
||||
}
|
||||
} else if ((state & ALTGR) && keymap->uses_altgr) {
|
||||
if (s < PS2_KEYMAP_SIZE)
|
||||
c = pgm_read_byte(keymap->altgr + s);
|
||||
} else if (state & (SHIFT_L | SHIFT_R)) {
|
||||
if (s < PS2_KEYMAP_SIZE)
|
||||
c = pgm_read_byte(keymap->shift + s);
|
||||
} else {
|
||||
if (s < PS2_KEYMAP_SIZE)
|
||||
c = pgm_read_byte(keymap->noshift + s);
|
||||
}
|
||||
state &= ~(BREAK | MODIFIER);
|
||||
if (c) return c;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
bool PS2Keyboard::available() {
|
||||
if (CharBuffer || UTF8next) return true;
|
||||
CharBuffer = get_iso8859_code();
|
||||
if (CharBuffer) return true;
|
||||
return false;
|
||||
}
|
||||
|
||||
int PS2Keyboard::read() {
|
||||
uint8_t result;
|
||||
|
||||
result = UTF8next;
|
||||
if (result) {
|
||||
UTF8next = 0;
|
||||
} else {
|
||||
result = CharBuffer;
|
||||
if (result) {
|
||||
CharBuffer = 0;
|
||||
} else {
|
||||
result = get_iso8859_code();
|
||||
}
|
||||
if (result >= 128) {
|
||||
UTF8next = (result & 0x3F) | 0x80;
|
||||
result = ((result >> 6) & 0x1F) | 0xC0;
|
||||
}
|
||||
}
|
||||
if (!result) return -1;
|
||||
return result;
|
||||
}
|
||||
|
||||
int PS2Keyboard::readUnicode() {
|
||||
int result;
|
||||
|
||||
result = CharBuffer;
|
||||
if (!result) result = get_iso8859_code();
|
||||
if (!result) return -1;
|
||||
UTF8next = 0;
|
||||
CharBuffer = 0;
|
||||
return result;
|
||||
}
|
||||
|
||||
PS2Keyboard::PS2Keyboard() {
|
||||
// nothing to do here, begin() does it all
|
||||
}
|
||||
|
||||
void PS2Keyboard::begin(uint8_t data_pin, uint8_t irq_pin, const PS2Keymap_t &map) {
|
||||
uint8_t irq_num=255;
|
||||
|
||||
DataPin = data_pin;
|
||||
keymap = ↦
|
||||
|
||||
// initialize the pins
|
||||
#ifdef INPUT_PULLUP
|
||||
pinMode(irq_pin, INPUT_PULLUP);
|
||||
pinMode(data_pin, INPUT_PULLUP);
|
||||
#else
|
||||
pinMode(irq_pin, INPUT);
|
||||
digitalWrite(irq_pin, HIGH);
|
||||
pinMode(data_pin, INPUT);
|
||||
digitalWrite(data_pin, HIGH);
|
||||
#endif
|
||||
|
||||
#ifdef CORE_INT_EVERY_PIN
|
||||
irq_num = irq_pin;
|
||||
|
||||
#else
|
||||
switch(irq_pin) {
|
||||
#ifdef CORE_INT0_PIN
|
||||
case CORE_INT0_PIN:
|
||||
irq_num = 0;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT1_PIN
|
||||
case CORE_INT1_PIN:
|
||||
irq_num = 1;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT2_PIN
|
||||
case CORE_INT2_PIN:
|
||||
irq_num = 2;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT3_PIN
|
||||
case CORE_INT3_PIN:
|
||||
irq_num = 3;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT4_PIN
|
||||
case CORE_INT4_PIN:
|
||||
irq_num = 4;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT5_PIN
|
||||
case CORE_INT5_PIN:
|
||||
irq_num = 5;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT6_PIN
|
||||
case CORE_INT6_PIN:
|
||||
irq_num = 6;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT7_PIN
|
||||
case CORE_INT7_PIN:
|
||||
irq_num = 7;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT8_PIN
|
||||
case CORE_INT8_PIN:
|
||||
irq_num = 8;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT9_PIN
|
||||
case CORE_INT9_PIN:
|
||||
irq_num = 9;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT10_PIN
|
||||
case CORE_INT10_PIN:
|
||||
irq_num = 10;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT11_PIN
|
||||
case CORE_INT11_PIN:
|
||||
irq_num = 11;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT12_PIN
|
||||
case CORE_INT12_PIN:
|
||||
irq_num = 12;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT13_PIN
|
||||
case CORE_INT13_PIN:
|
||||
irq_num = 13;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT14_PIN
|
||||
case CORE_INT14_PIN:
|
||||
irq_num = 14;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT15_PIN
|
||||
case CORE_INT15_PIN:
|
||||
irq_num = 15;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT16_PIN
|
||||
case CORE_INT16_PIN:
|
||||
irq_num = 16;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT17_PIN
|
||||
case CORE_INT17_PIN:
|
||||
irq_num = 17;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT18_PIN
|
||||
case CORE_INT18_PIN:
|
||||
irq_num = 18;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT19_PIN
|
||||
case CORE_INT19_PIN:
|
||||
irq_num = 19;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT20_PIN
|
||||
case CORE_INT20_PIN:
|
||||
irq_num = 20;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT21_PIN
|
||||
case CORE_INT21_PIN:
|
||||
irq_num = 21;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT22_PIN
|
||||
case CORE_INT22_PIN:
|
||||
irq_num = 22;
|
||||
break;
|
||||
#endif
|
||||
#ifdef CORE_INT23_PIN
|
||||
case CORE_INT23_PIN:
|
||||
irq_num = 23;
|
||||
break;
|
||||
#endif
|
||||
}
|
||||
#endif
|
||||
|
||||
head = 0;
|
||||
tail = 0;
|
||||
if (irq_num < 255) {
|
||||
attachInterrupt(irq_num, ps2interrupt, FALLING);
|
||||
}
|
||||
}
|
||||
|
||||
|
|
@ -1,212 +0,0 @@
|
|||
/*
|
||||
PS2Keyboard.h - PS2Keyboard library
|
||||
Copyright (c) 2007 Free Software Foundation. All right reserved.
|
||||
Written by Christian Weichel <info@32leaves.net>
|
||||
|
||||
** Mostly rewritten Paul Stoffregen <paul@pjrc.com>, June 2010
|
||||
** Modified for use with Arduino 13 by L. Abraham Smith, <n3bah@microcompdesign.com> *
|
||||
** Modified for easy interrup pin assignement on method begin(datapin,irq_pin). Cuningan <cuninganreset@gmail.com> **
|
||||
|
||||
This library is free software; you can redistribute it and/or
|
||||
modify it under the terms of the GNU Lesser General Public
|
||||
License as published by the Free Software Foundation; either
|
||||
version 2.1 of the License, or (at your option) any later version.
|
||||
|
||||
This library is distributed in the hope that it will be useful,
|
||||
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
||||
Lesser General Public License for more details.
|
||||
|
||||
You should have received a copy of the GNU Lesser General Public
|
||||
License along with this library; if not, write to the Free Software
|
||||
Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
|
||||
*/
|
||||
|
||||
|
||||
#ifndef PS2Keyboard_h
|
||||
#define PS2Keyboard_h
|
||||
|
||||
#if defined(ARDUINO) && ARDUINO >= 100
|
||||
#include "Arduino.h" // for attachInterrupt, FALLING
|
||||
#else
|
||||
#include "WProgram.h"
|
||||
#endif
|
||||
|
||||
#include "utility/int_pins.h"
|
||||
|
||||
// Every call to read() returns a single byte for each
|
||||
// keystroke. These configure what byte will be returned
|
||||
// for each "special" key. To ignore a key, use zero.
|
||||
#define PS2_TAB 9
|
||||
#define PS2_ENTER 13
|
||||
#define PS2_BACKSPACE 127
|
||||
#define PS2_ESC 27
|
||||
#define PS2_INSERT 0
|
||||
#define PS2_DELETE 127
|
||||
#define PS2_HOME 0
|
||||
#define PS2_END 0
|
||||
#define PS2_PAGEUP 25
|
||||
#define PS2_PAGEDOWN 26
|
||||
#define PS2_UPARROW 11
|
||||
#define PS2_LEFTARROW 8
|
||||
#define PS2_DOWNARROW 10
|
||||
#define PS2_RIGHTARROW 21
|
||||
#define PS2_F1 0
|
||||
#define PS2_F2 0
|
||||
#define PS2_F3 0
|
||||
#define PS2_F4 0
|
||||
#define PS2_F5 0
|
||||
#define PS2_F6 0
|
||||
#define PS2_F7 0
|
||||
#define PS2_F8 0
|
||||
#define PS2_F9 0
|
||||
#define PS2_F10 0
|
||||
#define PS2_F11 0
|
||||
#define PS2_F12 0
|
||||
#define PS2_SCROLL 0
|
||||
|
||||
#define PS2_INVERTED_EXCLAMATION 161 // ¡
|
||||
#define PS2_CENT_SIGN 162 // ¢
|
||||
#define PS2_POUND_SIGN 163 // £
|
||||
#define PS2_CURRENCY_SIGN 164 // ¤
|
||||
#define PS2_YEN_SIGN 165 // ¥
|
||||
#define PS2_BROKEN_BAR 166 // ¦
|
||||
#define PS2_SECTION_SIGN 167 // §
|
||||
#define PS2_DIAERESIS 168 // ¨
|
||||
#define PS2_COPYRIGHT_SIGN 169 // ©
|
||||
#define PS2_FEMININE_ORDINAL 170 // ª
|
||||
#define PS2_LEFT_DOUBLE_ANGLE_QUOTE 171 // «
|
||||
#define PS2_NOT_SIGN 172 // ¬
|
||||
#define PS2_HYPHEN 173
|
||||
#define PS2_REGISTERED_SIGN 174 // ®
|
||||
#define PS2_MACRON 175 // ¯
|
||||
#define PS2_DEGREE_SIGN 176 // °
|
||||
#define PS2_PLUS_MINUS_SIGN 177 // ±
|
||||
#define PS2_SUPERSCRIPT_TWO 178 // ²
|
||||
#define PS2_SUPERSCRIPT_THREE 179 // ³
|
||||
#define PS2_ACUTE_ACCENT 180 // ´
|
||||
#define PS2_MICRO_SIGN 181 // µ
|
||||
#define PS2_PILCROW_SIGN 182 // ¶
|
||||
#define PS2_MIDDLE_DOT 183 // ·
|
||||
#define PS2_CEDILLA 184 // ¸
|
||||
#define PS2_SUPERSCRIPT_ONE 185 // ¹
|
||||
#define PS2_MASCULINE_ORDINAL 186 // º
|
||||
#define PS2_RIGHT_DOUBLE_ANGLE_QUOTE 187 // »
|
||||
#define PS2_FRACTION_ONE_QUARTER 188 // ¼
|
||||
#define PS2_FRACTION_ONE_HALF 189 // ½
|
||||
#define PS2_FRACTION_THREE_QUARTERS 190 // ¾
|
||||
#define PS2_INVERTED_QUESTION MARK 191 // ¿
|
||||
#define PS2_A_GRAVE 192 // À
|
||||
#define PS2_A_ACUTE 193 // Á
|
||||
#define PS2_A_CIRCUMFLEX 194 // Â
|
||||
#define PS2_A_TILDE 195 // Ã
|
||||
#define PS2_A_DIAERESIS 196 // Ä
|
||||
#define PS2_A_RING_ABOVE 197 // Å
|
||||
#define PS2_AE 198 // Æ
|
||||
#define PS2_C_CEDILLA 199 // Ç
|
||||
#define PS2_E_GRAVE 200 // È
|
||||
#define PS2_E_ACUTE 201 // É
|
||||
#define PS2_E_CIRCUMFLEX 202 // Ê
|
||||
#define PS2_E_DIAERESIS 203 // Ë
|
||||
#define PS2_I_GRAVE 204 // Ì
|
||||
#define PS2_I_ACUTE 205 // Í
|
||||
#define PS2_I_CIRCUMFLEX 206 // Î
|
||||
#define PS2_I_DIAERESIS 207 // Ï
|
||||
#define PS2_ETH 208 // Ð
|
||||
#define PS2_N_TILDE 209 // Ñ
|
||||
#define PS2_O_GRAVE 210 // Ò
|
||||
#define PS2_O_ACUTE 211 // Ó
|
||||
#define PS2_O_CIRCUMFLEX 212 // Ô
|
||||
#define PS2_O_TILDE 213 // Õ
|
||||
#define PS2_O_DIAERESIS 214 // Ö
|
||||
#define PS2_MULTIPLICATION 215 // ×
|
||||
#define PS2_O_STROKE 216 // Ø
|
||||
#define PS2_U_GRAVE 217 // Ù
|
||||
#define PS2_U_ACUTE 218 // Ú
|
||||
#define PS2_U_CIRCUMFLEX 219 // Û
|
||||
#define PS2_U_DIAERESIS 220 // Ü
|
||||
#define PS2_Y_ACUTE 221 // Ý
|
||||
#define PS2_THORN 222 // Þ
|
||||
#define PS2_SHARP_S 223 // ß
|
||||
#define PS2_a_GRAVE 224 // à
|
||||
#define PS2_a_ACUTE 225 // á
|
||||
#define PS2_a_CIRCUMFLEX 226 // â
|
||||
#define PS2_a_TILDE 227 // ã
|
||||
#define PS2_a_DIAERESIS 228 // ä
|
||||
#define PS2_a_RING_ABOVE 229 // å
|
||||
#define PS2_ae 230 // æ
|
||||
#define PS2_c_CEDILLA 231 // ç
|
||||
#define PS2_e_GRAVE 232 // è
|
||||
#define PS2_e_ACUTE 233 // é
|
||||
#define PS2_e_CIRCUMFLEX 234 // ê
|
||||
#define PS2_e_DIAERESIS 235 // ë
|
||||
#define PS2_i_GRAVE 236 // ì
|
||||
#define PS2_i_ACUTE 237 // í
|
||||
#define PS2_i_CIRCUMFLEX 238 // î
|
||||
#define PS2_i_DIAERESIS 239 // ï
|
||||
#define PS2_eth 240 // ð
|
||||
#define PS2_n_TILDE 241 // ñ
|
||||
#define PS2_o_GRAVE 242 // ò
|
||||
#define PS2_o_ACUTE 243 // ó
|
||||
#define PS2_o_CIRCUMFLEX 244 // ô
|
||||
#define PS2_o_TILDE 245 // õ
|
||||
#define PS2_o_DIAERESIS 246 // ö
|
||||
#define PS2_DIVISION 247 // ÷
|
||||
#define PS2_o_STROKE 248 // ø
|
||||
#define PS2_u_GRAVE 249 // ù
|
||||
#define PS2_u_ACUTE 250 // ú
|
||||
#define PS2_u_CIRCUMFLEX 251 // û
|
||||
#define PS2_u_DIAERESIS 252 // ü
|
||||
#define PS2_y_ACUTE 253 // ý
|
||||
#define PS2_thorn 254 // þ
|
||||
#define PS2_y_DIAERESIS 255 // ÿ
|
||||
|
||||
|
||||
#define PS2_KEYMAP_SIZE 136
|
||||
|
||||
typedef struct {
|
||||
uint8_t noshift[PS2_KEYMAP_SIZE];
|
||||
uint8_t shift[PS2_KEYMAP_SIZE];
|
||||
uint8_t uses_altgr;
|
||||
uint8_t altgr[PS2_KEYMAP_SIZE];
|
||||
} PS2Keymap_t;
|
||||
|
||||
|
||||
extern const PROGMEM PS2Keymap_t PS2Keymap_US;
|
||||
extern const PROGMEM PS2Keymap_t PS2Keymap_German;
|
||||
extern const PROGMEM PS2Keymap_t PS2Keymap_French;
|
||||
|
||||
|
||||
/**
|
||||
* Purpose: Provides an easy access to PS2 keyboards
|
||||
* Author: Christian Weichel
|
||||
*/
|
||||
class PS2Keyboard {
|
||||
public:
|
||||
/**
|
||||
* This constructor does basically nothing. Please call the begin(int,int)
|
||||
* method before using any other method of this class.
|
||||
*/
|
||||
PS2Keyboard();
|
||||
|
||||
/**
|
||||
* Starts the keyboard "service" by registering the external interrupt.
|
||||
* setting the pin modes correctly and driving those needed to high.
|
||||
* The propably best place to call this method is in the setup routine.
|
||||
*/
|
||||
static void begin(uint8_t dataPin, uint8_t irq_pin, const PS2Keymap_t &map = PS2Keymap_US);
|
||||
|
||||
/**
|
||||
* Returns true if there is a char to be read, false if not.
|
||||
*/
|
||||
static bool available();
|
||||
|
||||
/**
|
||||
* Returns the char last read from the keyboard.
|
||||
* If there is no char available, -1 is returned.
|
||||
*/
|
||||
static int read();
|
||||
static int readUnicode();
|
||||
};
|
||||
|
||||
#endif
|
|
@ -1,7 +0,0 @@
|
|||
#PS/2 Keyboard Library#
|
||||
|
||||
PS2Keyboard allows you to use a keyboard for user input.
|
||||
|
||||
http://www.pjrc.com/teensy/td_libs_PS2Keyboard.html
|
||||
|
||||
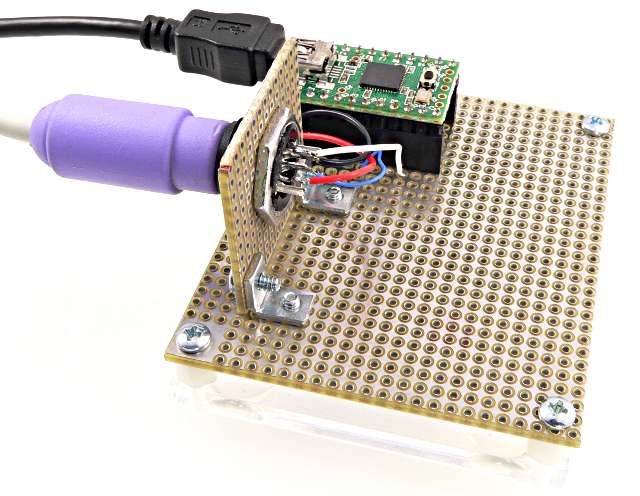
|
|
@ -1,26 +0,0 @@
|
|||
#######################################
|
||||
# Syntax Coloring Map For PS2Keyboard
|
||||
#######################################
|
||||
|
||||
#######################################
|
||||
# Datatypes (KEYWORD1)
|
||||
#######################################
|
||||
|
||||
PS2Keyboard KEYWORD1
|
||||
|
||||
#######################################
|
||||
# Methods and Functions (KEYWORD2)
|
||||
#######################################
|
||||
|
||||
#######################################
|
||||
# Constants (LITERAL1)
|
||||
#######################################
|
||||
|
||||
PS2_KC_BREAK LITERAL1
|
||||
PS2_KC_ENTER LITERAL1
|
||||
PS2_KC_ESC LITERAL1
|
||||
PS2_KC_KPLUS LITERAL1
|
||||
PS2_KC_KMINUS LITERAL1
|
||||
PS2_KC_KMULTI LITERAL1
|
||||
PS2_KC_NUM LITERAL1
|
||||
PS2_KC_BKSP LITERAL1
|
|
@ -1,16 +0,0 @@
|
|||
{
|
||||
"name": "PS2Keyboard",
|
||||
"keywords": "keyboard, emulation",
|
||||
"description": "PS2Keyboard (PS/2) allows you to use a keyboard for user input",
|
||||
"repository":
|
||||
{
|
||||
"type": "git",
|
||||
"url": "https://github.com/PaulStoffregen/PS2Keyboard.git"
|
||||
},
|
||||
"frameworks": "arduino",
|
||||
"platforms":
|
||||
[
|
||||
"atmelavr",
|
||||
"teensy"
|
||||
]
|
||||
}
|
|
@ -1,10 +0,0 @@
|
|||
name=PS2Keyboard
|
||||
version=2.4
|
||||
author=Christian Weichel, Paul Stoffregen, L. Abraham Smith, Cuningan
|
||||
maintainer=Paul Stoffregen
|
||||
sentence=Use a PS/2 Keyboard for input
|
||||
paragraph=
|
||||
category=Signal Input/Output
|
||||
url=https://github.com/PaulStoffregen/PS2Keyboard
|
||||
architectures=*
|
||||
|
|
@ -1,65 +0,0 @@
|
|||
// interrupt pins for known boards
|
||||
|
||||
// Teensy and maybe others automatically define this info
|
||||
#if !defined(CORE_INT0_PIN) && !defined(CORE_INT1_PIN) && !defined(CORE_INT2_PIN)&& !defined(CORE_INT3_PIN)
|
||||
|
||||
// Arduino Mega
|
||||
#if defined(__AVR_ATmega1280__) || defined(__AVR_ATmega2560__) // Arduino Mega
|
||||
#define CORE_INT0_PIN 2
|
||||
#define CORE_INT1_PIN 3
|
||||
#define CORE_INT2_PIN 21
|
||||
#define CORE_INT3_PIN 20
|
||||
#define CORE_INT4_PIN 19
|
||||
#define CORE_INT5_PIN 18
|
||||
|
||||
// Arduino Due (untested)
|
||||
#elif defined(__SAM3X8E__)
|
||||
#define CORE_INT_EVERY_PIN
|
||||
#ifndef PROGMEM
|
||||
#define PROGMEM
|
||||
#endif
|
||||
#ifndef pgm_read_byte
|
||||
#define pgm_read_byte(addr) (*(const unsigned char *)(addr))
|
||||
#endif
|
||||
|
||||
// Arduino Leonardo (untested)
|
||||
#elif defined(__AVR_ATmega32U4__) && !defined(CORE_TEENSY)
|
||||
#define CORE_INT0_PIN 3
|
||||
#define CORE_INT1_PIN 2
|
||||
#define CORE_INT2_PIN 0
|
||||
#define CORE_INT3_PIN 1
|
||||
|
||||
// Sanguino (untested)
|
||||
#elif defined(__AVR_ATmega644P__) || defined(__AVR_ATmega644__) // Sanguino
|
||||
#define CORE_INT0_PIN 10
|
||||
#define CORE_INT1_PIN 11
|
||||
#define CORE_INT2_PIN 2
|
||||
|
||||
// Chipkit Uno32 (untested)
|
||||
#elif defined(__PIC32MX__) && defined(_BOARD_UNO_)
|
||||
#define CORE_INT0_PIN 38
|
||||
#define CORE_INT1_PIN 2
|
||||
#define CORE_INT2_PIN 7
|
||||
#define CORE_INT3_PIN 8
|
||||
#define CORE_INT4_PIN 35
|
||||
|
||||
// Chipkit Mega32 (untested)
|
||||
#elif defined(__PIC32MX__) && defined(_BOARD_MEGA_)
|
||||
#define CORE_INT0_PIN 3
|
||||
#define CORE_INT1_PIN 2
|
||||
#define CORE_INT2_PIN 7
|
||||
#define CORE_INT3_PIN 21
|
||||
#define CORE_INT4_PIN 20
|
||||
|
||||
// http://hlt.media.mit.edu/?p=1229
|
||||
#elif defined(__AVR_ATtiny45__) || defined(__AVR_ATtiny85__)
|
||||
#define CORE_INT0_PIN 2
|
||||
|
||||
// Arduino Uno, Duemilanove, LilyPad, Mini, Fio, etc...
|
||||
#else
|
||||
#define CORE_INT0_PIN 2
|
||||
#define CORE_INT1_PIN 3
|
||||
|
||||
#endif
|
||||
#endif
|
||||
|
|
@ -1,34 +0,0 @@
|
|||
# Arduino-TVout
|
||||
|
||||
# Hosted here to keep it available from Arduino IDE (and for simple download as the original Google Code has been archived).
|
||||
|
||||
This is a library for generating composite video on an ATmega microcontroller.
|
||||
|
||||
This branch of the TVout library has been patched to allow use with the Arduino Leonardo. The goal of this project is to create a simple interrupt driven library for generating composite video on a single AVR chip.
|
||||
|
||||
Currently the output is NTSC or PAL at a resolution of 128x96 by default. The library currently works on ATmega168,328,1280,2560,644p,1284p,32U4,AT90USB1286 and more can be added by editing spec/hardware_setup.h.
|
||||
|
||||
There are some timing issues with the m1284p, may be related to sanguino core.
|
||||
|
||||
MCU | SYNC | VIDEO | AUDIO | Arduino | SYNC | VIDEO | AUDIO
|
||||
---|---|---|---|---|---|---|---
|
||||
m168,m328 | B 1 | D 7 | B 3 | NG,Decimila,UNO | 9 | 7 | 11
|
||||
m1280,m2560 | B 5 | A 7 | B 4 | Mega | 11 | A7(D29) | 10
|
||||
m644,m1284p | D 5 | A 7 | D 7 | sanguino | 13 | A7(D24) | 8
|
||||
m32u4 | B 5 | B 4 | B 7 | Leonardo | 9 | 8 | 11
|
||||
AT90USB1286 | B 5 | F 7 | B 4 | -- | -- | -- | --
|
||||
|
||||
## Connections
|
||||
|
||||
SYNC is on OCR1A and AUDIO is on OCR2A (except on the Arduino Leonardo, where AUDIO is on OCR0A)
|
||||
|
||||
There are some timing issues with the m1284p, may be related to sanguino core.
|
||||
|
||||
On NG, Decimila, UNO and Nano the sync is pin 9, video on 7 and audio on 11. On Mega2560 sync is pin 11, video is on A7(D29) and audio is on pin 10.
|
||||
|
||||
|
||||
## Examples
|
||||
|
||||
https://youtu.be/MEg_V4YZDh0
|
||||
|
||||
https://youtu.be/bHpFv_x_8Kk
|
|
@ -1,158 +0,0 @@
|
|||
/*
|
||||
Copyright (c) 2010 Myles Metzer
|
||||
Permission is hereby granted, free of charge, to any person
|
||||
obtaining a copy of this software and associated documentation
|
||||
files (the "Software"), to deal in the Software without
|
||||
restriction, including without limitation the rights to use,
|
||||
copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the
|
||||
Software is furnished to do so, subject to the following
|
||||
conditions:
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
|
||||
OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
|
||||
HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
|
||||
WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
|
||||
FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
|
||||
OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#ifndef TVOUT_H
|
||||
#define TVOUT_H
|
||||
|
||||
#include <Arduino.h>
|
||||
#include "video_gen.h"
|
||||
#include "fontALL.h"
|
||||
|
||||
#define PAL 1
|
||||
#define NTSC 0
|
||||
#define _PAL 1
|
||||
#define _NTSC 0
|
||||
|
||||
#define WHITE 1
|
||||
#define BLACK 0
|
||||
#define INVERT 2
|
||||
|
||||
#define UP 0
|
||||
#define DOWN 1
|
||||
#define LEFT 2
|
||||
#define RIGHT 3
|
||||
|
||||
#define DEC 10
|
||||
#define HEX 16
|
||||
#define OCT 8
|
||||
#define BIN 2
|
||||
#define BYTE 0
|
||||
|
||||
class TVout {
|
||||
public:
|
||||
uint8_t* screen;
|
||||
uint8_t begin(uint8_t mode);
|
||||
uint8_t begin(uint8_t mode, uint8_t x, uint8_t y);
|
||||
void end();
|
||||
|
||||
// accessor functions
|
||||
unsigned int hres();
|
||||
unsigned int vres();
|
||||
char charLine();
|
||||
|
||||
// flow control functions
|
||||
void delay(unsigned int x);
|
||||
void delayFrame(unsigned int x);
|
||||
unsigned long millis();
|
||||
|
||||
// basic rendering functions
|
||||
void clearScreen();
|
||||
void invert();
|
||||
void fill(uint8_t color);
|
||||
void setPixel(uint8_t x, uint8_t y, char c);
|
||||
bool getPixel(uint8_t x, uint8_t y);
|
||||
void drawLine(uint8_t x0, uint8_t y0, uint8_t x1, uint8_t y1, char c);
|
||||
void drawRow(uint8_t line, uint16_t x0, uint16_t x1, uint8_t c);
|
||||
void drawColumn(uint8_t row, uint16_t y0, uint16_t y1, uint8_t c);
|
||||
void drawRect(uint8_t x0, uint8_t y0, uint8_t w, uint8_t h, char c, char fc = -1);
|
||||
void drawCircle(uint8_t x0, uint8_t y0, uint8_t radius, char c, char fc = -1);
|
||||
void bitmap(uint8_t x, uint8_t y, const unsigned char* bmp, uint16_t i = 0, uint8_t width = 0, uint8_t lines = 0);
|
||||
void shift(uint8_t distance, uint8_t direction);
|
||||
|
||||
// printing functions
|
||||
void printChar(uint8_t x, uint8_t y, unsigned char c);
|
||||
void setCursor(uint8_t x, uint8_t y);
|
||||
void selectFont(const unsigned char* f);
|
||||
|
||||
void write(uint8_t);
|
||||
void write(const char* str);
|
||||
void write(const uint8_t* buffer, uint8_t size);
|
||||
|
||||
void print(const char[]);
|
||||
void print(char, int = BYTE);
|
||||
void print(unsigned char, int = BYTE);
|
||||
void print(int, int = DEC);
|
||||
void print(unsigned int, int = DEC);
|
||||
void print(long, int = DEC);
|
||||
void print(unsigned long, int = DEC);
|
||||
void print(float, int = 2);
|
||||
|
||||
void print(uint8_t, uint8_t, const char[]);
|
||||
void print(uint8_t, uint8_t, char, int = BYTE);
|
||||
void print(uint8_t, uint8_t, unsigned char, int = BYTE);
|
||||
void print(uint8_t, uint8_t, int, int = DEC);
|
||||
void print(uint8_t, uint8_t, unsigned int, int = DEC);
|
||||
void print(uint8_t, uint8_t, long, int = DEC);
|
||||
void print(uint8_t, uint8_t, unsigned long, int = DEC);
|
||||
void print(uint8_t, uint8_t, float, int = 2);
|
||||
|
||||
void println(uint8_t, uint8_t, const char[]);
|
||||
void println(uint8_t, uint8_t, char, int = BYTE);
|
||||
void println(uint8_t, uint8_t, unsigned char, int = BYTE);
|
||||
void println(uint8_t, uint8_t, int, int = DEC);
|
||||
void println(uint8_t, uint8_t, unsigned int, int = DEC);
|
||||
void println(uint8_t, uint8_t, long, int = DEC);
|
||||
void println(uint8_t, uint8_t, unsigned long, int = DEC);
|
||||
void println(uint8_t, uint8_t, float, int = 2);
|
||||
void println(uint8_t, uint8_t);
|
||||
|
||||
void println(const char[]);
|
||||
void println(char, int = BYTE);
|
||||
void println(unsigned char, int = BYTE);
|
||||
void println(int, int = DEC);
|
||||
void println(unsigned int, int = DEC);
|
||||
void println(long, int = DEC);
|
||||
void println(unsigned long, int = DEC);
|
||||
void println(float, int = 2);
|
||||
void println(void);
|
||||
|
||||
void printPGM(const char[]);
|
||||
void printPGM(uint8_t, uint8_t, const char[]);
|
||||
|
||||
void printNumber(unsigned long, uint8_t);
|
||||
void printFloat(float, uint8_t);
|
||||
|
||||
// tone functions
|
||||
void tone(unsigned int frequency, unsigned long durationMS);
|
||||
void tone(unsigned int frequency);
|
||||
void noTone();
|
||||
|
||||
uint8_t _cursorX, _cursorY;
|
||||
const unsigned char* font;
|
||||
|
||||
private:
|
||||
// hook setup functions
|
||||
void setVBIHook(void (*func)());
|
||||
void setHBIHook(void (*func)());
|
||||
|
||||
// override setup functions
|
||||
void forceVscale(char sfactor);
|
||||
void forceOutStart(uint8_t time);
|
||||
void forceLineStart(uint8_t line);
|
||||
void incTxtLine();
|
||||
|
||||
|
||||
|
||||
};
|
||||
|
||||
static void inline sp(uint8_t x, uint8_t y, char c);
|
||||
#endif
|
|
@ -1,671 +0,0 @@
|
|||
#include "font4x6.h"
|
||||
|
||||
PROGMEM const unsigned char font4x6[] = {
|
||||
4,6,32,
|
||||
//space
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//!
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//"
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//#
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//$
|
||||
0b01000000,
|
||||
0b01100000,
|
||||
0b11000000,
|
||||
0b01100000,
|
||||
0b11000000,
|
||||
0b00000000,
|
||||
//%
|
||||
0b10100000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//&
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b11000000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//'
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//(
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//)
|
||||
0b10000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//*
|
||||
0b01000000,
|
||||
0b10100000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//+
|
||||
0b00000000,
|
||||
0b01000000,
|
||||
0b11100000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//-
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//.
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
// /
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//0
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//1
|
||||
0b01000000,
|
||||
0b11000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//2
|
||||
0b11100000,
|
||||
0b00100000,
|
||||
0b11100000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//3
|
||||
0b11100000,
|
||||
0b00100000,
|
||||
0b11100000,
|
||||
0b00100000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//4
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
//5
|
||||
0b11100000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b00100000,
|
||||
0b11000000,
|
||||
0b00000000,
|
||||
//6
|
||||
0b11000000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//7
|
||||
0b11100000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//8
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//9
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b00100000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
//:
|
||||
0b00000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//;
|
||||
0b00000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//<
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b01000000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
//=
|
||||
0b00000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//>
|
||||
0b10000000,
|
||||
0b01000000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//?
|
||||
0b11000000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//@
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//A
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//B
|
||||
0b11000000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b11000000,
|
||||
0b00000000,
|
||||
//C
|
||||
0b11100000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//D
|
||||
0b11000000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b11000000,
|
||||
0b00000000,
|
||||
//E
|
||||
0b11100000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//F
|
||||
0b11100000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//G
|
||||
0b11100000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//H
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//I
|
||||
0b11100000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//J
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//K
|
||||
0b10000000,
|
||||
0b10100000,
|
||||
0b11000000,
|
||||
0b11000000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//L
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//M
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//N
|
||||
0b11000000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//O
|
||||
0b01000000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//P
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//Q
|
||||
0b01000000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b01100110,
|
||||
0b00000000,
|
||||
//R
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b11000000,
|
||||
0b11100000,
|
||||
0b10101010,
|
||||
0b00000000,
|
||||
//S
|
||||
0b11100000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b00100000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//T
|
||||
0b11100000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//U
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//V
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//W
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b11100000,
|
||||
0b10100010,
|
||||
0b00000000,
|
||||
//X
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b01000000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//Y
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//Z
|
||||
0b11100000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//[
|
||||
0b11000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b11000000,
|
||||
0b00000000,
|
||||
0, //for the life of me I have no idea why this is needed....
|
||||
//\
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b01000000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
//]
|
||||
0b11000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b11000000,
|
||||
0b00000000,
|
||||
//^
|
||||
0b01000000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//_
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//`
|
||||
0b10000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//a
|
||||
0b00000000,
|
||||
0b01100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
//b
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b11000000,
|
||||
0b10100000,
|
||||
0b11000000,
|
||||
0b00000000,
|
||||
//c
|
||||
0b00000000,
|
||||
0b01100000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
//d
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b01100000,
|
||||
0b10100000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
//e
|
||||
0b01000000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b10000000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
//f
|
||||
0b01100000,
|
||||
0b01000000,
|
||||
0b11100000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//g
|
||||
0b01100000,
|
||||
0b10100000,
|
||||
0b01100000,
|
||||
0b00100000,
|
||||
0b11000000,
|
||||
0b00000000,
|
||||
//h
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b11000000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//i
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//j
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b11000000,
|
||||
0b00000000,
|
||||
//k
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10100000,
|
||||
0b11000000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//l
|
||||
0b11000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//m
|
||||
0b00000000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//n
|
||||
0b00000000,
|
||||
0b11000000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//o
|
||||
0b00000000,
|
||||
0b01000000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//p
|
||||
0b00000000,
|
||||
0b11000000,
|
||||
0b10100000,
|
||||
0b11000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//q
|
||||
0b00000000,
|
||||
0b01100000,
|
||||
0b10100000,
|
||||
0b01100000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
//r
|
||||
0b00000000,
|
||||
0b11000000,
|
||||
0b10100000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//s
|
||||
0b00000000,
|
||||
0b01100000,
|
||||
0b01000000,
|
||||
0b00100000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
//t
|
||||
0b01000000,
|
||||
0b11100000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//u
|
||||
0b00000000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
//v
|
||||
0b00000000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//w
|
||||
0b00000000,
|
||||
0b10100000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//x
|
||||
0b00000000,
|
||||
0b10100000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b10100000,
|
||||
0b00000000,
|
||||
//y
|
||||
0b00000000,
|
||||
0b10100000,
|
||||
0b11100000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//x
|
||||
0b00000000,
|
||||
0b11100000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
//{
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b11000000,
|
||||
0b01000000,
|
||||
0b00100010,
|
||||
0b00000000,
|
||||
//|
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//}
|
||||
0b10000000,
|
||||
0b01000000,
|
||||
0b01100000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//~
|
||||
0b00000000,
|
||||
0b10100000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000
|
||||
};
|
|
@ -1,7 +0,0 @@
|
|||
#ifndef FONT4X6_h
|
||||
#define FONT4X6_h
|
||||
#include <avr/pgmspace.h>
|
||||
|
||||
extern const unsigned char font4x6[];
|
||||
|
||||
#endif
|
|
@ -1,870 +0,0 @@
|
|||
#include "font6x8.h"
|
||||
|
||||
PROGMEM const unsigned char font6x8[] = {
|
||||
|
||||
6,8,32,
|
||||
//32 Space
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//33 Exclamation !
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//34 Quotes "
|
||||
0b01010000,
|
||||
0b01010000,
|
||||
0b01010000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//35 Number #
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b01010000,
|
||||
0b11111000,
|
||||
0b01010000,
|
||||
0b11111000,
|
||||
0b01010000,
|
||||
0b00000000,
|
||||
//36 Dollars $
|
||||
0b00100000,
|
||||
0b01110000,
|
||||
0b10100000,
|
||||
0b01110000,
|
||||
0b00101000,
|
||||
0b01110000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
//37 Percent %
|
||||
0b00000000,
|
||||
0b11001000,
|
||||
0b11010000,
|
||||
0b00100000,
|
||||
0b01011000,
|
||||
0b10011000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//38 Ampersand &
|
||||
0b00100000,
|
||||
0b01010000,
|
||||
0b10000000,
|
||||
0b01000000,
|
||||
0b10101000,
|
||||
0b10010000,
|
||||
0b01101000,
|
||||
0b00000000,
|
||||
//39 Single Quote '
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//40 Left Parenthesis (
|
||||
0b00010000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00100000,
|
||||
0b00010000,
|
||||
0b00000000,
|
||||
//41 Right Parenthesis )
|
||||
0b01000000,
|
||||
0b00100000,
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//42 Star *
|
||||
0b00010000,
|
||||
0b00111000,
|
||||
0b00010000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//43 Plus +
|
||||
0b00000000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b11111000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//44 Comma ,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b00000000,
|
||||
//45 Minus -
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b11111000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//46 Period .
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00010000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
// 47 Backslash /
|
||||
0b00000000,
|
||||
0b00001000,
|
||||
0b00010000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
// 48 Zero
|
||||
0b01110000,
|
||||
0b10001000,
|
||||
0b10101000,
|
||||
0b10101000,
|
||||
0b10001000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//49 One
|
||||
0b00100000,
|
||||
0b01100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//50 two
|
||||
0b01110000,
|
||||
0b10001000,
|
||||
0b00010000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b11111000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//51 Three
|
||||
0b11111000,
|
||||
0b00010000,
|
||||
0b00100000,
|
||||
0b00010000,
|
||||
0b10001000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//52 Four
|
||||
0b10010000,
|
||||
0b10010000,
|
||||
0b10010000,
|
||||
0b11111000,
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//53 Five
|
||||
0b11111000,
|
||||
0b10000000,
|
||||
0b11110000,
|
||||
0b00001000,
|
||||
0b10001000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//54 Six
|
||||
0b01110000,
|
||||
0b10000000,
|
||||
0b11110000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//55 Seven
|
||||
0b11111000,
|
||||
0b00001000,
|
||||
0b00010000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//56 Eight
|
||||
0b01110000,
|
||||
0b10001000,
|
||||
0b01110000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//57 Nine
|
||||
0b01110000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b01111000,
|
||||
0b00001000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//58 :
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//59 ;
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
//60 <
|
||||
0b00000000,
|
||||
0b00011000,
|
||||
0b01100000,
|
||||
0b10000000,
|
||||
0b01100000,
|
||||
0b00011000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//61 =
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b01111000,
|
||||
0b00000000,
|
||||
0b01111000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//62 >
|
||||
0b00000000,
|
||||
0b11000000,
|
||||
0b00110000,
|
||||
0b00001000,
|
||||
0b00110000,
|
||||
0b11000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//63 ?
|
||||
0b01100000,
|
||||
0b10010000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//64 @
|
||||
0b01110000,
|
||||
0b10001000,
|
||||
0b10011000,
|
||||
0b10101000,
|
||||
0b10010000,
|
||||
0b10001000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
//65 A
|
||||
0b00100000,
|
||||
0b01010000,
|
||||
0b10001000,
|
||||
0b11111000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//B
|
||||
0b11110000,
|
||||
0b10001000,
|
||||
0b11110000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b11110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//C
|
||||
0b01110000,
|
||||
0b10001000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10001000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//D
|
||||
0b11110000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b11110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//E
|
||||
0b11111000,
|
||||
0b10000000,
|
||||
0b11111000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b11111000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//F
|
||||
0b11111000,
|
||||
0b10000000,
|
||||
0b11110000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//G
|
||||
0b01110000,
|
||||
0b10001000,
|
||||
0b10000000,
|
||||
0b10011000,
|
||||
0b10001000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//H
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b11111000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//I
|
||||
0b01110000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//J
|
||||
0b00111000,
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b10010000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//K
|
||||
0b10001000,
|
||||
0b10010000,
|
||||
0b11100000,
|
||||
0b10100000,
|
||||
0b10010000,
|
||||
0b10001000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//L
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b11111000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//M
|
||||
0b10001000,
|
||||
0b11011000,
|
||||
0b10101000,
|
||||
0b10101000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//N
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b11001000,
|
||||
0b10101000,
|
||||
0b10011000,
|
||||
0b10001000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//O
|
||||
0b01110000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//P
|
||||
0b11110000,
|
||||
0b10001000,
|
||||
0b11110000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//Q
|
||||
0b01110000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10101000,
|
||||
0b10010000,
|
||||
0b01101000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//R
|
||||
0b11110000,
|
||||
0b10001000,
|
||||
0b11110000,
|
||||
0b10100000,
|
||||
0b10010000,
|
||||
0b10001000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//S
|
||||
0b01111000,
|
||||
0b10000000,
|
||||
0b01110000,
|
||||
0b00001000,
|
||||
0b00001000,
|
||||
0b11110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//T
|
||||
0b11111000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//U
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//V
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b01010000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//W
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10101000,
|
||||
0b10101000,
|
||||
0b10101000,
|
||||
0b01010000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//X
|
||||
0b10001000,
|
||||
0b01010000,
|
||||
0b00100000,
|
||||
0b01010000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//Y
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b01010000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//Z
|
||||
0b11111000,
|
||||
0b00001000,
|
||||
0b00010000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b11111000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//91 [
|
||||
0b11100000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//92 (backslash)
|
||||
0b00000000,
|
||||
0b10000000,
|
||||
0b01000000,
|
||||
0b00100000,
|
||||
0b00010000,
|
||||
0b00001000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//93 ]
|
||||
0b00111000,
|
||||
0b00001000,
|
||||
0b00001000,
|
||||
0b00001000,
|
||||
0b00001000,
|
||||
0b00111000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//94 ^
|
||||
0b00100000,
|
||||
0b01010000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//95 _
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b11111000,
|
||||
0b00000000,
|
||||
//96 `
|
||||
0b10000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//97 a
|
||||
0b00000000,
|
||||
0b01100000,
|
||||
0b00010000,
|
||||
0b01110000,
|
||||
0b10010000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//98 b
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b10010000,
|
||||
0b10010000,
|
||||
0b11100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//99 c
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b01110000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
// 100 d
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b01110000,
|
||||
0b10010000,
|
||||
0b10010000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//101 e
|
||||
0b00000000,
|
||||
0b01100000,
|
||||
0b10010000,
|
||||
0b11110000,
|
||||
0b10000000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//102 f
|
||||
0b00110000,
|
||||
0b01000000,
|
||||
0b11100000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//103 g
|
||||
0b00000000,
|
||||
0b01100000,
|
||||
0b10010000,
|
||||
0b01110000,
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
//104 h
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b11100000,
|
||||
0b10010000,
|
||||
0b10010000,
|
||||
0b10010000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//105 i
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//106 j
|
||||
0b00010000,
|
||||
0b00000000,
|
||||
0b00110000,
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
//107 k
|
||||
0b10000000,
|
||||
0b10010000,
|
||||
0b10100000,
|
||||
0b11000000,
|
||||
0b10100000,
|
||||
0b10010000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//108 l
|
||||
0b01100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b01110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//109 m
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b01010000,
|
||||
0b10101000,
|
||||
0b10101000,
|
||||
0b10101000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//110 n
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b11110000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//111 o
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b01100000,
|
||||
0b10010000,
|
||||
0b10010000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//112 p
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b01100000,
|
||||
0b10010000,
|
||||
0b11110000,
|
||||
0b10000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//113 q
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b01100000,
|
||||
0b10010000,
|
||||
0b11110000,
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b00000000,
|
||||
//114 r
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b10110000,
|
||||
0b01001000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//115 s
|
||||
0b00000000,
|
||||
0b00110000,
|
||||
0b01000000,
|
||||
0b00100000,
|
||||
0b00010000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//116 t
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b11100000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
// 117u
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b10010000,
|
||||
0b10010000,
|
||||
0b10010000,
|
||||
0b01100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//118 v
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b10001000,
|
||||
0b10001000,
|
||||
0b01010000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//119 w
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b10001000,
|
||||
0b10101000,
|
||||
0b10101000,
|
||||
0b01010000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//120 x
|
||||
0b00000000,
|
||||
0b10001000,
|
||||
0b01010000,
|
||||
0b00100000,
|
||||
0b01010000,
|
||||
0b10001000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//121 y
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b10010000,
|
||||
0b10010000,
|
||||
0b01100000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b00000000,
|
||||
//122 z
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b11110000,
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b11110000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//123 {
|
||||
0b00100000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b10000000,
|
||||
0b01000000,
|
||||
0b01000000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
//124 |
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
//125 }
|
||||
0b00100000,
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b00001000,
|
||||
0b00010000,
|
||||
0b00010000,
|
||||
0b00100000,
|
||||
0b00000000,
|
||||
//126 ~
|
||||
0b01000000,
|
||||
0b10101000,
|
||||
0b00010000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
//127 DEL
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000,
|
||||
0b00000000
|
||||
};
|
|
@ -1,8 +0,0 @@
|
|||
#ifndef FONT6X8_H
|
||||
#define FONT6X8_H
|
||||
|
||||
#include <avr/pgmspace.h>
|
||||
|
||||
extern const unsigned char font6x8[];
|
||||
|
||||
#endif
|
|
@ -1,133 +0,0 @@
|
|||
#include "font8x8.h"
|
||||
|
||||
PROGMEM const unsigned char font8x8[] = {
|
||||
8,8,0,
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
||||
0x00, 0x3E, 0x41, 0x55, 0x41, 0x55, 0x49, 0x3E,
|
||||
0x00, 0x3E, 0x7F, 0x6B, 0x7F, 0x6B, 0x77, 0x3E,
|
||||
0x00, 0x22, 0x77, 0x7F, 0x7F, 0x3E, 0x1C, 0x08,
|
||||
0x00, 0x08, 0x1C, 0x3E, 0x7F, 0x3E, 0x1C, 0x08,
|
||||
0x00, 0x08, 0x1C, 0x2A, 0x7F, 0x2A, 0x08, 0x1C,
|
||||
0x00, 0x08, 0x1C, 0x3E, 0x7F, 0x3E, 0x08, 0x1C,
|
||||
0x00, 0x00, 0x1C, 0x3E, 0x3E, 0x3E, 0x1C, 0x00,
|
||||
0xFF, 0xFF, 0xE3, 0xC1, 0xC1, 0xC1, 0xE3, 0xFF,
|
||||
0x00, 0x00, 0x1C, 0x22, 0x22, 0x22, 0x1C, 0x00,
|
||||
0xFF, 0xFF, 0xE3, 0xDD, 0xDD, 0xDD, 0xE3, 0xFF,
|
||||
0x00, 0x0F, 0x03, 0x05, 0x39, 0x48, 0x48, 0x30,
|
||||
0x00, 0x08, 0x3E, 0x08, 0x1C, 0x22, 0x22, 0x1C,
|
||||
0x00, 0x18, 0x14, 0x10, 0x10, 0x30, 0x70, 0x60,
|
||||
0x00, 0x0F, 0x19, 0x11, 0x13, 0x37, 0x76, 0x60,
|
||||
0x00, 0x08, 0x2A, 0x1C, 0x77, 0x1C, 0x2A, 0x08,
|
||||
0x00, 0x60, 0x78, 0x7E, 0x7F, 0x7E, 0x78, 0x60,
|
||||
0x00, 0x03, 0x0F, 0x3F, 0x7F, 0x3F, 0x0F, 0x03,
|
||||
0x00, 0x08, 0x1C, 0x2A, 0x08, 0x2A, 0x1C, 0x08,
|
||||
0x00, 0x66, 0x66, 0x66, 0x66, 0x00, 0x66, 0x66,
|
||||
0x00, 0x3F, 0x65, 0x65, 0x3D, 0x05, 0x05, 0x05,
|
||||
0x00, 0x0C, 0x32, 0x48, 0x24, 0x12, 0x4C, 0x30,
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x7F, 0x7F, 0x7F,
|
||||
0x00, 0x08, 0x1C, 0x2A, 0x08, 0x2A, 0x1C, 0x3E,
|
||||
0x00, 0x08, 0x1C, 0x3E, 0x7F, 0x1C, 0x1C, 0x1C,
|
||||
0x00, 0x1C, 0x1C, 0x1C, 0x7F, 0x3E, 0x1C, 0x08,
|
||||
0x00, 0x08, 0x0C, 0x7E, 0x7F, 0x7E, 0x0C, 0x08,
|
||||
0x00, 0x08, 0x18, 0x3F, 0x7F, 0x3F, 0x18, 0x08,
|
||||
0x00, 0x00, 0x00, 0x70, 0x70, 0x70, 0x7F, 0x7F,
|
||||
0x00, 0x00, 0x14, 0x22, 0x7F, 0x22, 0x14, 0x00,
|
||||
0x00, 0x08, 0x1C, 0x1C, 0x3E, 0x3E, 0x7F, 0x7F,
|
||||
0x00, 0x7F, 0x7F, 0x3E, 0x3E, 0x1C, 0x1C, 0x08,
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
||||
0x00, 0x18, 0x3C, 0x3C, 0x18, 0x18, 0x00, 0x18,
|
||||
0x00, 0x36, 0x36, 0x14, 0x00, 0x00, 0x00, 0x00,
|
||||
0x00, 0x36, 0x36, 0x7F, 0x36, 0x7F, 0x36, 0x36,
|
||||
0x00, 0x08, 0x1E, 0x20, 0x1C, 0x02, 0x3C, 0x08,
|
||||
0x00, 0x60, 0x66, 0x0C, 0x18, 0x30, 0x66, 0x06,
|
||||
0x00, 0x3C, 0x66, 0x3C, 0x28, 0x65, 0x66, 0x3F,
|
||||
0x00, 0x18, 0x18, 0x18, 0x30, 0x00, 0x00, 0x00,
|
||||
0x00, 0x60, 0x30, 0x18, 0x18, 0x18, 0x30, 0x60,
|
||||
0x00, 0x06, 0x0C, 0x18, 0x18, 0x18, 0x0C, 0x06,
|
||||
0x00, 0x00, 0x36, 0x1C, 0x7F, 0x1C, 0x36, 0x00,
|
||||
0x00, 0x00, 0x08, 0x08, 0x3E, 0x08, 0x08, 0x00,
|
||||
0x00, 0x00, 0x00, 0x00, 0x30, 0x30, 0x30, 0x60,
|
||||
0x00, 0x00, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x00,
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x60, 0x60,
|
||||
0x00, 0x00, 0x06, 0x0C, 0x18, 0x30, 0x60, 0x00,
|
||||
0x00, 0x3C, 0x66, 0x6E, 0x76, 0x66, 0x66, 0x3C,
|
||||
0x00, 0x18, 0x18, 0x38, 0x18, 0x18, 0x18, 0x7E,
|
||||
0x00, 0x3C, 0x66, 0x06, 0x0C, 0x30, 0x60, 0x7E,
|
||||
0x00, 0x3C, 0x66, 0x06, 0x1C, 0x06, 0x66, 0x3C,
|
||||
0x00, 0x0C, 0x1C, 0x2C, 0x4C, 0x7E, 0x0C, 0x0C,
|
||||
0x00, 0x7E, 0x60, 0x7C, 0x06, 0x06, 0x66, 0x3C,
|
||||
0x00, 0x3C, 0x66, 0x60, 0x7C, 0x66, 0x66, 0x3C,
|
||||
0x00, 0x7E, 0x66, 0x0C, 0x0C, 0x18, 0x18, 0x18,
|
||||
0x00, 0x3C, 0x66, 0x66, 0x3C, 0x66, 0x66, 0x3C,
|
||||
0x00, 0x3C, 0x66, 0x66, 0x3E, 0x06, 0x66, 0x3C,
|
||||
0x00, 0x00, 0x18, 0x18, 0x00, 0x18, 0x18, 0x00,
|
||||
0x00, 0x00, 0x18, 0x18, 0x00, 0x18, 0x18, 0x30,
|
||||
0x00, 0x06, 0x0C, 0x18, 0x30, 0x18, 0x0C, 0x06,
|
||||
0x00, 0x00, 0x00, 0x3C, 0x00, 0x3C, 0x00, 0x00,
|
||||
0x00, 0x60, 0x30, 0x18, 0x0C, 0x18, 0x30, 0x60,
|
||||
0x00, 0x3C, 0x66, 0x06, 0x1C, 0x18, 0x00, 0x18,
|
||||
0x00, 0x38, 0x44, 0x5C, 0x58, 0x42, 0x3C, 0x00,
|
||||
0x00, 0x3C, 0x66, 0x66, 0x7E, 0x66, 0x66, 0x66,
|
||||
0x00, 0x7C, 0x66, 0x66, 0x7C, 0x66, 0x66, 0x7C,
|
||||
0x00, 0x3C, 0x66, 0x60, 0x60, 0x60, 0x66, 0x3C,
|
||||
0x00, 0x7C, 0x66, 0x66, 0x66, 0x66, 0x66, 0x7C,
|
||||
0x00, 0x7E, 0x60, 0x60, 0x7C, 0x60, 0x60, 0x7E,
|
||||
0x00, 0x7E, 0x60, 0x60, 0x7C, 0x60, 0x60, 0x60,
|
||||
0x00, 0x3C, 0x66, 0x60, 0x60, 0x6E, 0x66, 0x3C,
|
||||
0x00, 0x66, 0x66, 0x66, 0x7E, 0x66, 0x66, 0x66,
|
||||
0x00, 0x3C, 0x18, 0x18, 0x18, 0x18, 0x18, 0x3C,
|
||||
0x00, 0x1E, 0x0C, 0x0C, 0x0C, 0x6C, 0x6C, 0x38,
|
||||
0x00, 0x66, 0x6C, 0x78, 0x70, 0x78, 0x6C, 0x66,
|
||||
0x00, 0x60, 0x60, 0x60, 0x60, 0x60, 0x60, 0x7E,
|
||||
0x00, 0x63, 0x77, 0x7F, 0x6B, 0x63, 0x63, 0x63,
|
||||
0x00, 0x63, 0x73, 0x7B, 0x6F, 0x67, 0x63, 0x63,
|
||||
0x00, 0x3C, 0x66, 0x66, 0x66, 0x66, 0x66, 0x3C,
|
||||
0x00, 0x7C, 0x66, 0x66, 0x66, 0x7C, 0x60, 0x60,
|
||||
0x00, 0x3C, 0x66, 0x66, 0x66, 0x6E, 0x3C, 0x06,
|
||||
0x00, 0x7C, 0x66, 0x66, 0x7C, 0x78, 0x6C, 0x66,
|
||||
0x00, 0x3C, 0x66, 0x60, 0x3C, 0x06, 0x66, 0x3C,
|
||||
0x00, 0x7E, 0x5A, 0x18, 0x18, 0x18, 0x18, 0x18,
|
||||
0x00, 0x66, 0x66, 0x66, 0x66, 0x66, 0x66, 0x3E,
|
||||
0x00, 0x66, 0x66, 0x66, 0x66, 0x66, 0x3C, 0x18,
|
||||
0x00, 0x63, 0x63, 0x63, 0x6B, 0x7F, 0x77, 0x63,
|
||||
0x00, 0x63, 0x63, 0x36, 0x1C, 0x36, 0x63, 0x63,
|
||||
0x00, 0x66, 0x66, 0x66, 0x3C, 0x18, 0x18, 0x18,
|
||||
0x00, 0x7E, 0x06, 0x0C, 0x18, 0x30, 0x60, 0x7E,
|
||||
0x00, 0x1E, 0x18, 0x18, 0x18, 0x18, 0x18, 0x1E,
|
||||
0x00, 0x00, 0x60, 0x30, 0x18, 0x0C, 0x06, 0x00,
|
||||
0x00, 0x78, 0x18, 0x18, 0x18, 0x18, 0x18, 0x78,
|
||||
0x00, 0x08, 0x14, 0x22, 0x41, 0x00, 0x00, 0x00,
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7F,
|
||||
0x00, 0x0C, 0x0C, 0x06, 0x00, 0x00, 0x00, 0x00,
|
||||
0x00, 0x00, 0x00, 0x3C, 0x06, 0x3E, 0x66, 0x3E,
|
||||
0x00, 0x60, 0x60, 0x60, 0x7C, 0x66, 0x66, 0x7C,
|
||||
0x00, 0x00, 0x00, 0x3C, 0x66, 0x60, 0x66, 0x3C,
|
||||
0x00, 0x06, 0x06, 0x06, 0x3E, 0x66, 0x66, 0x3E,
|
||||
0x00, 0x00, 0x00, 0x3C, 0x66, 0x7E, 0x60, 0x3C,
|
||||
0x00, 0x1C, 0x36, 0x30, 0x30, 0x7C, 0x30, 0x30,
|
||||
0x00, 0x00, 0x3E, 0x66, 0x66, 0x3E, 0x06, 0x3C,
|
||||
0x00, 0x60, 0x60, 0x60, 0x7C, 0x66, 0x66, 0x66,
|
||||
0x00, 0x00, 0x18, 0x00, 0x18, 0x18, 0x18, 0x3C,
|
||||
0x00, 0x0C, 0x00, 0x0C, 0x0C, 0x6C, 0x6C, 0x38,
|
||||
0x00, 0x60, 0x60, 0x66, 0x6C, 0x78, 0x6C, 0x66,
|
||||
0x00, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18,
|
||||
0x00, 0x00, 0x00, 0x63, 0x77, 0x7F, 0x6B, 0x6B,
|
||||
0x00, 0x00, 0x00, 0x7C, 0x7E, 0x66, 0x66, 0x66,
|
||||
0x00, 0x00, 0x00, 0x3C, 0x66, 0x66, 0x66, 0x3C,
|
||||
0x00, 0x00, 0x7C, 0x66, 0x66, 0x7C, 0x60, 0x60,
|
||||
0x00, 0x00, 0x3C, 0x6C, 0x6C, 0x3C, 0x0D, 0x0F,
|
||||
0x00, 0x00, 0x00, 0x7C, 0x66, 0x66, 0x60, 0x60,
|
||||
0x00, 0x00, 0x00, 0x3E, 0x40, 0x3C, 0x02, 0x7C,
|
||||
0x00, 0x00, 0x18, 0x18, 0x7E, 0x18, 0x18, 0x18,
|
||||
0x00, 0x00, 0x00, 0x66, 0x66, 0x66, 0x66, 0x3E,
|
||||
0x00, 0x00, 0x00, 0x00, 0x66, 0x66, 0x3C, 0x18,
|
||||
0x00, 0x00, 0x00, 0x63, 0x6B, 0x6B, 0x6B, 0x3E,
|
||||
0x00, 0x00, 0x00, 0x66, 0x3C, 0x18, 0x3C, 0x66,
|
||||
0x00, 0x00, 0x00, 0x66, 0x66, 0x3E, 0x06, 0x3C,
|
||||
0x00, 0x00, 0x00, 0x3C, 0x0C, 0x18, 0x30, 0x3C,
|
||||
0x00, 0x0E, 0x18, 0x18, 0x30, 0x18, 0x18, 0x0E,
|
||||
0x00, 0x18, 0x18, 0x18, 0x00, 0x18, 0x18, 0x18,
|
||||
0x00, 0x70, 0x18, 0x18, 0x0C, 0x18, 0x18, 0x70,
|
||||
0x00, 0x00, 0x00, 0x3A, 0x6C, 0x00, 0x00, 0x00,
|
||||
0x00, 0x08, 0x1C, 0x36, 0x63, 0x41, 0x41, 0x7F
|
||||
};
|
|
@ -1,7 +0,0 @@
|
|||
#ifndef FONT8X8_H
|
||||
#define FONT8X8_H
|
||||
|
||||
#include <avr/pgmspace.h>
|
||||
extern const unsigned char font8x8[];
|
||||
|
||||
#endif
|
|
@ -1,261 +0,0 @@
|
|||
#include "font8x8ext.h"
|
||||
|
||||
PROGMEM const unsigned char font8x8ext[] = {
|
||||
8,8,0,
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
||||
0x7E, 0x81, 0xA5, 0x81, 0xBD, 0x99, 0x81, 0x7E,
|
||||
0x7E, 0xFF, 0xDB, 0xFF, 0xC3, 0xE7, 0xFF, 0x7E,
|
||||
0x6C, 0xFE, 0xFE, 0xFE, 0x7C, 0x38, 0x10, 0x00,
|
||||
0x10, 0x38, 0x7C, 0xFE, 0x7C, 0x38, 0x10, 0x00,
|
||||
0x38, 0x7C, 0x38, 0xFE, 0xFE, 0xD6, 0x10, 0x38,
|
||||
0x10, 0x38, 0x7C, 0xFE, 0xFE, 0x7C, 0x10, 0x38,
|
||||
0x00, 0x00, 0x18, 0x3C, 0x3C, 0x18, 0x00, 0x00,
|
||||
0xFF, 0xFF, 0xE7, 0xC3, 0xC3, 0xE7, 0xFF, 0xFF,
|
||||
0x00, 0x3C, 0x66, 0x42, 0x42, 0x66, 0x3C, 0x00,
|
||||
0xFF, 0xC3, 0x99, 0xBD, 0xBD, 0x99, 0xC3, 0xFF,
|
||||
0x0F, 0x07, 0x0F, 0x7D, 0xCC, 0xCC, 0xCC, 0x78,
|
||||
0x3C, 0x66, 0x66, 0x66, 0x3C, 0x18, 0x7E, 0x18,
|
||||
0x3F, 0x33, 0x3F, 0x30, 0x30, 0x70, 0xF0, 0xE0,
|
||||
0x7F, 0x63, 0x7F, 0x63, 0x63, 0x67, 0xE6, 0xC0,
|
||||
0x18, 0xDB, 0x3C, 0xE7, 0xE7, 0x3C, 0xDB, 0x18,
|
||||
0x80, 0xE0, 0xF8, 0xFE, 0xF8, 0xE0, 0x80, 0x00,
|
||||
0x02, 0x0E, 0x3E, 0xFE, 0x3E, 0x0E, 0x02, 0x00,
|
||||
0x18, 0x3C, 0x7E, 0x18, 0x18, 0x7E, 0x3C, 0x18,
|
||||
0x66, 0x66, 0x66, 0x66, 0x66, 0x00, 0x66, 0x00,
|
||||
0x7F, 0xDB, 0xDB, 0x7B, 0x1B, 0x1B, 0x1B, 0x00,
|
||||
0x3E, 0x61, 0x3C, 0x66, 0x66, 0x3C, 0x86, 0x7C,
|
||||
0x00, 0x00, 0x00, 0x00, 0x7E, 0x7E, 0x7E, 0x00,
|
||||
0x18, 0x3C, 0x7E, 0x18, 0x7E, 0x3C, 0x18, 0xFF,
|
||||
0x18, 0x3C, 0x7E, 0x18, 0x18, 0x18, 0x18, 0x00,
|
||||
0x18, 0x18, 0x18, 0x18, 0x7E, 0x3C, 0x18, 0x00,
|
||||
0x00, 0x18, 0x0C, 0xFE, 0x0C, 0x18, 0x00, 0x00,
|
||||
0x00, 0x30, 0x60, 0xFE, 0x60, 0x30, 0x00, 0x00,
|
||||
0x00, 0x00, 0xC0, 0xC0, 0xC0, 0xFE, 0x00, 0x00,
|
||||
0x00, 0x24, 0x66, 0xFF, 0x66, 0x24, 0x00, 0x00,
|
||||
0x00, 0x18, 0x3C, 0x7E, 0xFF, 0xFF, 0x00, 0x00,
|
||||
0x00, 0xFF, 0xFF, 0x7E, 0x3C, 0x18, 0x00, 0x00,
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
||||
0x18, 0x3C, 0x3C, 0x18, 0x18, 0x00, 0x18, 0x00,
|
||||
0x66, 0x66, 0x24, 0x00, 0x00, 0x00, 0x00, 0x00,
|
||||
0x6C, 0x6C, 0xFE, 0x6C, 0xFE, 0x6C, 0x6C, 0x00,
|
||||
0x18, 0x3E, 0x60, 0x3C, 0x06, 0x7C, 0x18, 0x00,
|
||||
0x00, 0xC6, 0xCC, 0x18, 0x30, 0x66, 0xC6, 0x00,
|
||||
0x38, 0x6C, 0x38, 0x76, 0xDC, 0xCC, 0x76, 0x00,
|
||||
0x18, 0x18, 0x30, 0x00, 0x00, 0x00, 0x00, 0x00,
|
||||
0x0C, 0x18, 0x30, 0x30, 0x30, 0x18, 0x0C, 0x00,
|
||||
0x30, 0x18, 0x0C, 0x0C, 0x0C, 0x18, 0x30, 0x00,
|
||||
0x00, 0x66, 0x3C, 0xFF, 0x3C, 0x66, 0x00, 0x00,
|
||||
0x00, 0x18, 0x18, 0x7E, 0x18, 0x18, 0x00, 0x00,
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x18, 0x18, 0x30,
|
||||
0x00, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x00, 0x00,
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x18, 0x18, 0x00,
|
||||
0x06, 0x0C, 0x18, 0x30, 0x60, 0xC0, 0x80, 0x00,
|
||||
0x7C, 0xC6, 0xCE, 0xD6, 0xE6, 0xC6, 0x7C, 0x00,
|
||||
0x18, 0x38, 0x18, 0x18, 0x18, 0x18, 0x7E, 0x00,
|
||||
0x7C, 0xC6, 0x06, 0x1C, 0x30, 0x66, 0xFE, 0x00,
|
||||
0x7C, 0xC6, 0x06, 0x3C, 0x06, 0xC6, 0x7C, 0x00,
|
||||
0x1C, 0x3C, 0x6C, 0xCC, 0xFE, 0x0C, 0x1E, 0x00,
|
||||
0xFE, 0xC0, 0xC0, 0xFC, 0x06, 0xC6, 0x7C, 0x00,
|
||||
0x38, 0x60, 0xC0, 0xFC, 0xC6, 0xC6, 0x7C, 0x00,
|
||||
0xFE, 0xC6, 0x0C, 0x18, 0x30, 0x30, 0x30, 0x00,
|
||||
0x7C, 0xC6, 0xC6, 0x7C, 0xC6, 0xC6, 0x7C, 0x00,
|
||||
0x7C, 0xC6, 0xC6, 0x7E, 0x06, 0x0C, 0x78, 0x00,
|
||||
0x00, 0x18, 0x18, 0x00, 0x00, 0x18, 0x18, 0x00,
|
||||
0x00, 0x18, 0x18, 0x00, 0x00, 0x18, 0x18, 0x30,
|
||||
0x06, 0x0C, 0x18, 0x30, 0x18, 0x0C, 0x06, 0x00,
|
||||
0x00, 0x00, 0x7E, 0x00, 0x00, 0x7E, 0x00, 0x00,
|
||||
0x60, 0x30, 0x18, 0x0C, 0x18, 0x30, 0x60, 0x00,
|
||||
0x7C, 0xC6, 0x0C, 0x18, 0x18, 0x00, 0x18, 0x00,
|
||||
0x7C, 0xC6, 0xDE, 0xDE, 0xDE, 0xC0, 0x78, 0x00,
|
||||
0x38, 0x6C, 0xC6, 0xFE, 0xC6, 0xC6, 0xC6, 0x00,
|
||||
0xFC, 0x66, 0x66, 0x7C, 0x66, 0x66, 0xFC, 0x00,
|
||||
0x3C, 0x66, 0xC0, 0xC0, 0xC0, 0x66, 0x3C, 0x00,
|
||||
0xF8, 0x6C, 0x66, 0x66, 0x66, 0x6C, 0xF8, 0x00,
|
||||
0xFE, 0x62, 0x68, 0x78, 0x68, 0x62, 0xFE, 0x00,
|
||||
0xFE, 0x62, 0x68, 0x78, 0x68, 0x60, 0xF0, 0x00,
|
||||
0x3C, 0x66, 0xC0, 0xC0, 0xCE, 0x66, 0x3A, 0x00,
|
||||
0xC6, 0xC6, 0xC6, 0xFE, 0xC6, 0xC6, 0xC6, 0x00,
|
||||
0x3C, 0x18, 0x18, 0x18, 0x18, 0x18, 0x3C, 0x00,
|
||||
0x1E, 0x0C, 0x0C, 0x0C, 0xCC, 0xCC, 0x78, 0x00,
|
||||
0xE6, 0x66, 0x6C, 0x78, 0x6C, 0x66, 0xE6, 0x00,
|
||||
0xF0, 0x60, 0x60, 0x60, 0x62, 0x66, 0xFE, 0x00,
|
||||
0xC6, 0xEE, 0xFE, 0xFE, 0xD6, 0xC6, 0xC6, 0x00,
|
||||
0xC6, 0xE6, 0xF6, 0xDE, 0xCE, 0xC6, 0xC6, 0x00,
|
||||
0x7C, 0xC6, 0xC6, 0xC6, 0xC6, 0xC6, 0x7C, 0x00,
|
||||
0xFC, 0x66, 0x66, 0x7C, 0x60, 0x60, 0xF0, 0x00,
|
||||
0x7C, 0xC6, 0xC6, 0xC6, 0xC6, 0xCE, 0x7C, 0x0E,
|
||||
0xFC, 0x66, 0x66, 0x7C, 0x6C, 0x66, 0xE6, 0x00,
|
||||
0x7C, 0xC6, 0x60, 0x38, 0x0C, 0xC6, 0x7C, 0x00,
|
||||
0x7E, 0x7E, 0x5A, 0x18, 0x18, 0x18, 0x3C, 0x00,
|
||||
0xC6, 0xC6, 0xC6, 0xC6, 0xC6, 0xC6, 0x7C, 0x00,
|
||||
0xC6, 0xC6, 0xC6, 0xC6, 0xC6, 0x6C, 0x38, 0x00,
|
||||
0xC6, 0xC6, 0xC6, 0xD6, 0xD6, 0xFE, 0x6C, 0x00,
|
||||
0xC6, 0xC6, 0x6C, 0x38, 0x6C, 0xC6, 0xC6, 0x00,
|
||||
0x66, 0x66, 0x66, 0x3C, 0x18, 0x18, 0x3C, 0x00,
|
||||
0xFE, 0xC6, 0x8C, 0x18, 0x32, 0x66, 0xFE, 0x00,
|
||||
0x3C, 0x30, 0x30, 0x30, 0x30, 0x30, 0x3C, 0x00,
|
||||
0xC0, 0x60, 0x30, 0x18, 0x0C, 0x06, 0x02, 0x00,
|
||||
0x3C, 0x0C, 0x0C, 0x0C, 0x0C, 0x0C, 0x3C, 0x00,
|
||||
0x10, 0x38, 0x6C, 0xC6, 0x00, 0x00, 0x00, 0x00,
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF,
|
||||
0x30, 0x18, 0x0C, 0x00, 0x00, 0x00, 0x00, 0x00,
|
||||
0x00, 0x00, 0x78, 0x0C, 0x7C, 0xCC, 0x76, 0x00,
|
||||
0xE0, 0x60, 0x7C, 0x66, 0x66, 0x66, 0xDC, 0x00,
|
||||
0x00, 0x00, 0x7C, 0xC6, 0xC0, 0xC6, 0x7C, 0x00,
|
||||
0x1C, 0x0C, 0x7C, 0xCC, 0xCC, 0xCC, 0x76, 0x00,
|
||||
0x00, 0x00, 0x7C, 0xC6, 0xFE, 0xC0, 0x7C, 0x00,
|
||||
0x3C, 0x66, 0x60, 0xF8, 0x60, 0x60, 0xF0, 0x00,
|
||||
0x00, 0x00, 0x76, 0xCC, 0xCC, 0x7C, 0x0C, 0xF8,
|
||||
0xE0, 0x60, 0x6C, 0x76, 0x66, 0x66, 0xE6, 0x00,
|
||||
0x18, 0x00, 0x38, 0x18, 0x18, 0x18, 0x3C, 0x00,
|
||||
0x06, 0x00, 0x06, 0x06, 0x06, 0x66, 0x66, 0x3C,
|
||||
0xE0, 0x60, 0x66, 0x6C, 0x78, 0x6C, 0xE6, 0x00,
|
||||
0x38, 0x18, 0x18, 0x18, 0x18, 0x18, 0x3C, 0x00,
|
||||
0x00, 0x00, 0xEC, 0xFE, 0xD6, 0xD6, 0xD6, 0x00,
|
||||
0x00, 0x00, 0xDC, 0x66, 0x66, 0x66, 0x66, 0x00,
|
||||
0x00, 0x00, 0x7C, 0xC6, 0xC6, 0xC6, 0x7C, 0x00,
|
||||
0x00, 0x00, 0xDC, 0x66, 0x66, 0x7C, 0x60, 0xF0,
|
||||
0x00, 0x00, 0x76, 0xCC, 0xCC, 0x7C, 0x0C, 0x1E,
|
||||
0x00, 0x00, 0xDC, 0x76, 0x60, 0x60, 0xF0, 0x00,
|
||||
0x00, 0x00, 0x7E, 0xC0, 0x7C, 0x06, 0xFC, 0x00,
|
||||
0x30, 0x30, 0xFC, 0x30, 0x30, 0x36, 0x1C, 0x00,
|
||||
0x00, 0x00, 0xCC, 0xCC, 0xCC, 0xCC, 0x76, 0x00,
|
||||
0x00, 0x00, 0xC6, 0xC6, 0xC6, 0x6C, 0x38, 0x00,
|
||||
0x00, 0x00, 0xC6, 0xD6, 0xD6, 0xFE, 0x6C, 0x00,
|
||||
0x00, 0x00, 0xC6, 0x6C, 0x38, 0x6C, 0xC6, 0x00,
|
||||
0x00, 0x00, 0xC6, 0xC6, 0xC6, 0x7E, 0x06, 0xFC,
|
||||
0x00, 0x00, 0x7E, 0x4C, 0x18, 0x32, 0x7E, 0x00,
|
||||
0x0E, 0x18, 0x18, 0x70, 0x18, 0x18, 0x0E, 0x00,
|
||||
0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x00,
|
||||
0x70, 0x18, 0x18, 0x0E, 0x18, 0x18, 0x70, 0x00,
|
||||
0x76, 0xDC, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
||||
0x00, 0x10, 0x38, 0x6C, 0xC6, 0xC6, 0xFE, 0x00,
|
||||
0x60, 0xF0, 0x60, 0x7C, 0x66, 0x66, 0x66, 0x0C,
|
||||
0xFC, 0x60, 0x7C, 0x66, 0x66, 0x66, 0x6C, 0x00,
|
||||
0x1C, 0x10, 0x00, 0x7E, 0x60, 0x60, 0x60, 0x00,
|
||||
0x38, 0x20, 0xFE, 0xC0, 0xC0, 0xC0, 0xC0, 0x00,
|
||||
0x6C, 0x00, 0x7C, 0xC6, 0xFE, 0xC0, 0x7C, 0x00,
|
||||
0x6C, 0xFE, 0x62, 0x78, 0x60, 0x62, 0xFE, 0x00,
|
||||
0x00, 0x00, 0x7C, 0xC6, 0xF0, 0xC6, 0x7C, 0x00,
|
||||
0x3C, 0x66, 0xC0, 0xF8, 0xC0, 0x66, 0x3C, 0x00,
|
||||
0x00, 0x00, 0x7E, 0xC0, 0x7C, 0x06, 0xFC, 0x00,
|
||||
0x7C, 0xC6, 0x60, 0x38, 0x0C, 0xC6, 0x7C, 0x00,
|
||||
0x30, 0x00, 0x30, 0x30, 0x30, 0x30, 0x78, 0x00,
|
||||
0x78, 0x30, 0x30, 0x30, 0x30, 0x30, 0x78, 0x00,
|
||||
0xCC, 0x00, 0x30, 0x30, 0x30, 0x30, 0x78, 0x00,
|
||||
0x66, 0x3C, 0x18, 0x18, 0x18, 0x18, 0x3C, 0x00,
|
||||
0x18, 0x00, 0x18, 0x18, 0x18, 0x18, 0x18, 0x70,
|
||||
0x1E, 0x0C, 0x0C, 0x0C, 0x0C, 0xCC, 0x78, 0x00,
|
||||
0x00, 0x00, 0x70, 0x50, 0x5C, 0x52, 0xDC, 0x00,
|
||||
0x70, 0x50, 0x5C, 0x52, 0x52, 0x52, 0xDC, 0x00,
|
||||
0x00, 0x00, 0xA0, 0xA0, 0xFC, 0xA2, 0xBC, 0x00,
|
||||
0xA0, 0xA0, 0xA0, 0xFC, 0xA2, 0xA2, 0xBC, 0x00,
|
||||
0x60, 0xF0, 0x60, 0x7C, 0x66, 0x66, 0x66, 0x00,
|
||||
0xF8, 0x60, 0x7C, 0x66, 0x66, 0x66, 0x66, 0x00,
|
||||
0x1C, 0x10, 0xC6, 0xD8, 0xF8, 0xCC, 0xC6, 0x00,
|
||||
0x18, 0xD6, 0xCC, 0xF8, 0xF8, 0xCC, 0xC6, 0x00,
|
||||
0x6C, 0x38, 0xC6, 0xC6, 0xC6, 0x7E, 0x06, 0x7C,
|
||||
0x38, 0xC6, 0xC6, 0x7E, 0x06, 0xC6, 0x7C, 0x00,
|
||||
0x00, 0x00, 0xC6, 0xC6, 0xC6, 0xC6, 0xFE, 0x10,
|
||||
0xC6, 0xC6, 0xC6, 0xC6, 0xC6, 0xC6, 0xFE, 0x10,
|
||||
0x00, 0x00, 0xDC, 0xD6, 0xF6, 0xD6, 0xDC, 0x00,
|
||||
0xDC, 0xD6, 0xD6, 0xF6, 0xD6, 0xD6, 0xDC, 0x00,
|
||||
0x00, 0x00, 0xF0, 0xB0, 0x3C, 0x36, 0x3C, 0x00,
|
||||
0xF0, 0xF0, 0xB0, 0x3C, 0x36, 0x36, 0x3C, 0x00,
|
||||
0x00, 0x00, 0x78, 0x0C, 0x7C, 0xCC, 0x76, 0x00,
|
||||
0x3E, 0x66, 0xC6, 0xC6, 0xFE, 0xC6, 0xC6, 0x00,
|
||||
0x06, 0x7C, 0xC0, 0x7C, 0xC6, 0xC6, 0x7C, 0x00,
|
||||
0xFE, 0x66, 0x60, 0x7C, 0x66, 0x66, 0xFC, 0x00,
|
||||
0x00, 0x00, 0xCC, 0xCC, 0xCC, 0xCC, 0xFE, 0x06,
|
||||
0xCC, 0xCC, 0xCC, 0xCC, 0xCC, 0xCC, 0xFE, 0x06,
|
||||
0x00, 0x00, 0x3C, 0x6C, 0x6C, 0x6C, 0xFE, 0xC6,
|
||||
0x1E, 0x36, 0x66, 0x66, 0x66, 0x66, 0xFF, 0xC3,
|
||||
0x00, 0x00, 0x7C, 0xC6, 0xFE, 0xC0, 0x7C, 0x00,
|
||||
0xFE, 0x62, 0x68, 0x78, 0x68, 0x62, 0xFE, 0x00,
|
||||
0x00, 0x00, 0x7C, 0xD6, 0xD6, 0x7C, 0x10, 0x38,
|
||||
0x7C, 0xD6, 0xD6, 0xD6, 0x7C, 0x10, 0x38, 0x00,
|
||||
0x00, 0x00, 0xFE, 0x66, 0x60, 0x60, 0xF0, 0x00,
|
||||
0xFE, 0x66, 0x60, 0x60, 0x60, 0x60, 0xF0, 0x00,
|
||||
0x00, 0x33, 0x66, 0xCC, 0x66, 0x33, 0x00, 0x00,
|
||||
0x00, 0xCC, 0x66, 0x33, 0x66, 0xCC, 0x00, 0x00,
|
||||
0x22, 0x88, 0x22, 0x88, 0x22, 0x88, 0x22, 0x88,
|
||||
0x55, 0xAA, 0x55, 0xAA, 0x55, 0xAA, 0x55, 0xAA,
|
||||
0xDB, 0x77, 0xDB, 0xEE, 0xDB, 0x77, 0xDB, 0xEE,
|
||||
0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18,
|
||||
0x18, 0x18, 0x18, 0x18, 0xF8, 0x18, 0x18, 0x18,
|
||||
0x00, 0x00, 0xC6, 0x6C, 0x38, 0x6C, 0xC6, 0x00,
|
||||
0xC6, 0xC6, 0x6C, 0x38, 0x6C, 0xC6, 0xC6, 0x00,
|
||||
0x00, 0x00, 0xC6, 0xCE, 0xDE, 0xF6, 0xE6, 0x00,
|
||||
0xC6, 0xC6, 0xCE, 0xDE, 0xF6, 0xE6, 0xC6, 0x00,
|
||||
0x36, 0x36, 0xF6, 0x06, 0xF6, 0x36, 0x36, 0x36,
|
||||
0x36, 0x36, 0x36, 0x36, 0x36, 0x36, 0x36, 0x36,
|
||||
0x00, 0x00, 0xFE, 0x06, 0xF6, 0x36, 0x36, 0x36,
|
||||
0x36, 0x36, 0xF6, 0x06, 0xFE, 0x00, 0x00, 0x00,
|
||||
0x00, 0x38, 0xC6, 0xCE, 0xDE, 0xF6, 0xE6, 0x00,
|
||||
0x38, 0xC6, 0xCE, 0xDE, 0xF6, 0xE6, 0xC6, 0x00,
|
||||
0x00, 0x00, 0x00, 0x00, 0xF8, 0x18, 0x18, 0x18,
|
||||
0x18, 0x18, 0x18, 0x18, 0x1F, 0x00, 0x00, 0x00,
|
||||
0x18, 0x18, 0x18, 0x18, 0xFF, 0x00, 0x00, 0x00,
|
||||
0x00, 0x00, 0x00, 0x00, 0xFF, 0x18, 0x18, 0x18,
|
||||
0x18, 0x18, 0x18, 0x18, 0x1F, 0x18, 0x18, 0x18,
|
||||
0x00, 0x00, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x00,
|
||||
0x18, 0x18, 0x18, 0x18, 0xFF, 0x18, 0x18, 0x18,
|
||||
0x00, 0x00, 0xE6, 0x6C, 0x78, 0x6C, 0xE6, 0x00,
|
||||
0xE6, 0x6C, 0x78, 0x78, 0x6C, 0x66, 0xE6, 0x00,
|
||||
0x36, 0x36, 0x37, 0x30, 0x3F, 0x00, 0x00, 0x00,
|
||||
0x00, 0x00, 0x3F, 0x30, 0x37, 0x36, 0x36, 0x36,
|
||||
0x36, 0x36, 0xF7, 0x00, 0xFF, 0x00, 0x00, 0x00,
|
||||
0x00, 0x00, 0xFF, 0x00, 0xF7, 0x36, 0x36, 0x36,
|
||||
0x36, 0x36, 0x37, 0x30, 0x37, 0x36, 0x36, 0x36,
|
||||
0x00, 0x00, 0xFF, 0x00, 0xFF, 0x00, 0x00, 0x00,
|
||||
0x36, 0x36, 0xF7, 0x00, 0xF7, 0x36, 0x36, 0x36,
|
||||
0x00, 0xC6, 0x7C, 0xC6, 0xC6, 0x7C, 0xC6, 0x00,
|
||||
0x00, 0x00, 0x3E, 0x66, 0x66, 0x66, 0xE6, 0x00,
|
||||
0x1E, 0x36, 0x66, 0x66, 0x66, 0x66, 0xC6, 0x00,
|
||||
0x00, 0x00, 0xC6, 0xFE, 0xFE, 0xD6, 0xC6, 0x00,
|
||||
0xC6, 0xEE, 0xFE, 0xFE, 0xD6, 0xC6, 0xC6, 0x00,
|
||||
0x00, 0x00, 0xC6, 0xC6, 0xFE, 0xC6, 0xC6, 0x00,
|
||||
0xC6, 0xC6, 0xC6, 0xFE, 0xC6, 0xC6, 0xC6, 0x00,
|
||||
0x00, 0x00, 0x7C, 0xC6, 0xC6, 0xC6, 0x7C, 0x00,
|
||||
0x7C, 0xC6, 0xC6, 0xC6, 0xC6, 0xC6, 0x7C, 0x00,
|
||||
0x00, 0x00, 0xFE, 0xC6, 0xC6, 0xC6, 0xC6, 0x00,
|
||||
0x18, 0x18, 0x18, 0x18, 0xF8, 0x00, 0x00, 0x00,
|
||||
0x00, 0x00, 0x00, 0x00, 0x1F, 0x18, 0x18, 0x18,
|
||||
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
|
||||
0x00, 0x00, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0xFF,
|
||||
0xFE, 0xC6, 0xC6, 0xC6, 0xC6, 0xC6, 0xC6, 0x00,
|
||||
0x00, 0x00, 0x7E, 0xC6, 0x7E, 0x66, 0xC6, 0x00,
|
||||
0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x00,
|
||||
0x7E, 0xC6, 0xC6, 0xC6, 0x7E, 0x66, 0xC6, 0x00,
|
||||
0x00, 0x00, 0xFC, 0x66, 0x66, 0x7C, 0x60, 0xF0,
|
||||
0xFC, 0x66, 0x66, 0x66, 0x7C, 0x60, 0xF0, 0x00,
|
||||
0x00, 0x00, 0x7C, 0xC6, 0xC0, 0xC6, 0x7C, 0x00,
|
||||
0x7C, 0xC6, 0xC0, 0xC0, 0xC0, 0xC6, 0x7C, 0x00,
|
||||
0x00, 0x00, 0x7E, 0x5A, 0x18, 0x18, 0x3C, 0x00,
|
||||
0x7E, 0x5A, 0x18, 0x18, 0x18, 0x18, 0x3C, 0x00,
|
||||
0x00, 0x00, 0xC6, 0xC6, 0xC6, 0x7E, 0x06, 0x7C,
|
||||
0xC6, 0xC6, 0xC6, 0x7E, 0x06, 0xC6, 0x7C, 0x00,
|
||||
0x00, 0x00, 0xD6, 0x7C, 0x38, 0x7C, 0xD6, 0x00,
|
||||
0xD6, 0xD6, 0x7C, 0x38, 0x7C, 0xD6, 0xD6, 0x00,
|
||||
0x00, 0x00, 0xFC, 0x66, 0x7C, 0x66, 0xFC, 0x00,
|
||||
0xFC, 0x66, 0x66, 0x7C, 0x66, 0x66, 0xFC, 0x00,
|
||||
0x00, 0x00, 0xF0, 0x60, 0x7C, 0x66, 0xFC, 0x00,
|
||||
0xF0, 0x60, 0x60, 0x7C, 0x66, 0x66, 0x7C, 0x00,
|
||||
0x8F, 0xCD, 0xEF, 0xFC, 0xDC, 0xCC, 0xCC, 0x00,
|
||||
0x00, 0x00, 0x00, 0x7C, 0x7C, 0x00, 0x00, 0x00,
|
||||
0x00, 0x00, 0xC6, 0xC6, 0xF6, 0xDE, 0xF6, 0x00,
|
||||
0xC6, 0xC6, 0xC6, 0xF6, 0xDE, 0xDE, 0xF6, 0x00,
|
||||
0x00, 0x00, 0x7C, 0xC6, 0x1C, 0xC6, 0x7C, 0x00,
|
||||
0x7C, 0xC6, 0x06, 0x3C, 0x06, 0xC6, 0x7C, 0x00,
|
||||
0x00, 0x00, 0xD6, 0xD6, 0xD6, 0xD6, 0xFE, 0x00,
|
||||
0xD6, 0xD6, 0xD6, 0xD6, 0xD6, 0xD6, 0xFE, 0x00,
|
||||
0x00, 0x00, 0x7C, 0xC6, 0x1E, 0xC6, 0x7C, 0x00,
|
||||
0x78, 0x8C, 0x06, 0x3E, 0x06, 0x8C, 0x78, 0x00,
|
||||
0x00, 0x00, 0xD6, 0xD6, 0xD6, 0xD6, 0xFF, 0x03,
|
||||
0xD6, 0xD6, 0xD6, 0xD6, 0xD6, 0xD6, 0xFF, 0x03,
|
||||
0x00, 0x00, 0xC6, 0xC6, 0x7E, 0x06, 0x06, 0x00,
|
||||
0xC6, 0xC6, 0xC6, 0x7E, 0x06, 0x06, 0x06, 0x00,
|
||||
0x3E, 0x61, 0x3C, 0x66, 0x66, 0x3C, 0x86, 0x7C,
|
||||
0x00, 0x00, 0x3C, 0x3C, 0x3C, 0x3C, 0x00, 0x00,
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
|
||||
};
|
|
@ -1,7 +0,0 @@
|
|||
#ifndef FONT8X8EXT_H
|
||||
#define FONT8X8EXT_H
|
||||
|
||||
#include <avr/pgmspace.h>
|
||||
extern const unsigned char font8x8ext[];
|
||||
|
||||
#endif
|
|
@ -1,9 +0,0 @@
|
|||
#ifndef FONTALL_H
|
||||
#define FONTALL_H
|
||||
|
||||
#include "font4x6.h"
|
||||
#include "font6x8.h"
|
||||
#include "font8x8.h"
|
||||
#include "font8x8ext.h"
|
||||
|
||||
#endif
|
|
@ -1,54 +0,0 @@
|
|||
NTSC LITERAL1
|
||||
PAL LITERAL1
|
||||
_NTSC LITERAL1
|
||||
_PAL LITERAL1
|
||||
WHITE LITERAL1
|
||||
BLACK LITERAL1
|
||||
INVERT LITERAL1
|
||||
UP LITERAL1
|
||||
DOWN LITERAL1
|
||||
LEFT LITERAL1
|
||||
RIGHT LITERAL1
|
||||
|
||||
TVout KEYWORD1
|
||||
|
||||
clearScreen KEYWORD2
|
||||
invert KEYWORD2
|
||||
|
||||
begin KEYWORD2
|
||||
end KEYWORD2
|
||||
force_vscale KEYWORD2
|
||||
force_outstart KEYWORD2
|
||||
force_linestart KEYWORD2
|
||||
hres KEYWORD2
|
||||
vres KEYWORD2
|
||||
charLine KEYWORD2
|
||||
fill KEYWORD2
|
||||
delay KEYWORD2
|
||||
delayFrame KEYWORD2
|
||||
millis KEYWORD2
|
||||
setPixel KEYWORD2
|
||||
getPixel KEYWORD2
|
||||
fill KEYWORD2
|
||||
shift KEYWORD2
|
||||
drawLine KEYWORD2
|
||||
drawRow KEYWORD2
|
||||
drawColumn KEYWORD2
|
||||
drawRect KEYWORD2
|
||||
drawCircle KEYWORD2
|
||||
bitmap KEYWORD2
|
||||
set_vbi_hook KEYWORD2
|
||||
set_hbi_hook KEYWORD2
|
||||
tone KEYWORD2
|
||||
noTone KEYWORD2
|
||||
printChar KEYWORD2
|
||||
setCursor KEYWORD2
|
||||
selectFont KEYWORD2
|
||||
print KEYWORD2
|
||||
println KEYWORD2
|
||||
printPGM KEYWORD2
|
||||
|
||||
font4x6 LITERAL1
|
||||
font6x8 LITERAL1
|
||||
font8x8 LITERAL1
|
||||
font8x8ext LITERAL1
|
|
@ -1,119 +0,0 @@
|
|||
#include "hardware_setup.h"
|
||||
|
||||
#ifndef ASM_MACROS_H
|
||||
#define ASM_MACROS_H
|
||||
|
||||
// delay macros
|
||||
__asm__ __volatile__ (
|
||||
// delay 1 clock cycle.
|
||||
".macro delay1\n\t"
|
||||
"nop\n"
|
||||
".endm\n"
|
||||
|
||||
// delay 2 clock cycles
|
||||
".macro delay2\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n"
|
||||
".endm\n"
|
||||
|
||||
// delay 3 clock cyles
|
||||
".macro delay3\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n"
|
||||
".endm\n"
|
||||
|
||||
// delay 4 clock cylces
|
||||
".macro delay4\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n"
|
||||
".endm\n"
|
||||
|
||||
// delay 5 clock cylces
|
||||
".macro delay5\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n"
|
||||
".endm\n"
|
||||
|
||||
// delay 6 clock cylces
|
||||
".macro delay6\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n"
|
||||
".endm\n"
|
||||
|
||||
// delay 7 clock cylces
|
||||
".macro delay7\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n"
|
||||
".endm\n"
|
||||
|
||||
// delay 8 clock cylces
|
||||
".macro delay8\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n"
|
||||
".endm\n"
|
||||
|
||||
// delay 9 clock cylces
|
||||
".macro delay9\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n"
|
||||
".endm\n"
|
||||
|
||||
// delay 10 clock cylces
|
||||
".macro delay10\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n\t"
|
||||
"nop\n"
|
||||
".endm\n"
|
||||
);
|
||||
|
||||
// common output macros, specific output macros at top of file
|
||||
__asm__ __volatile__ (
|
||||
|
||||
// save port 16 and clear the video bit
|
||||
".macro svprt p\n\t"
|
||||
"in r16,\\p\n\t"
|
||||
ANDI_HWS
|
||||
".endm\n"
|
||||
|
||||
// ouput 1 bit port safe
|
||||
".macro o1bs p\n\t"
|
||||
BLD_HWS
|
||||
"out \\p,r16\n"
|
||||
".endm\n"
|
||||
);
|
||||
#endif
|
|
@ -1,146 +0,0 @@
|
|||
// sound is output on OC2A
|
||||
// sync output is on OC1A
|
||||
|
||||
// ENABLE_FAST_OUTPUT chooses the highest bit of a port over the original output method
|
||||
// comment out this line to switch back to the original output pins.
|
||||
#define ENABLE_FAST_OUTPUT
|
||||
|
||||
#ifndef HARDWARE_SETUP_H
|
||||
#define HARDWARE_SETUP_H
|
||||
|
||||
// device specific settings.
|
||||
#if defined(__AVR_ATmega1280__) || defined(__AVR_ATmega1281__) || defined(__AVR_ATmega2560__) || defined(__AVR_ATmega2561__)
|
||||
#if defined(ENABLE_FAST_OUTPUT)
|
||||
#define PORT_VID PORTA
|
||||
#define DDR_VID DDRA
|
||||
#define VID_PIN 7
|
||||
#else
|
||||
//video
|
||||
#define PORT_VID PORTB
|
||||
#define DDR_VID DDRB
|
||||
#define VID_PIN 6
|
||||
#endif
|
||||
//sync
|
||||
#define PORT_SYNC PORTB
|
||||
#define DDR_SYNC DDRB
|
||||
#define SYNC_PIN 5
|
||||
//sound
|
||||
#define PORT_SND PORTB
|
||||
#define DDR_SND DDRB
|
||||
#define SND_PIN 4
|
||||
|
||||
#elif defined(__AVR_ATmega644__) || defined(__AVR_ATmega644P__) || defined(__AVR_ATmega1284__) || defined(__AVR_ATmega1284P__)
|
||||
//video
|
||||
#if defined(ENABLE_FAST_OUTPUT)
|
||||
#define PORT_VID PORTA
|
||||
#define DDR_VID DDRA
|
||||
#define VID_PIN 7
|
||||
#else
|
||||
#define PORT_VID PORTD
|
||||
#define DDR_VID DDRD
|
||||
#define VID_PIN 4
|
||||
#endif
|
||||
//sync
|
||||
#define PORT_SYNC PORTD
|
||||
#define DDR_SYNC DDRD
|
||||
#define SYNC_PIN 5
|
||||
//sound
|
||||
#define PORT_SND PORTD
|
||||
#define DDR_SND DDRD
|
||||
#define SND_PIN 7
|
||||
|
||||
// Arduino UNO
|
||||
#elif defined(__AVR_ATmega8__) || defined(__AVR_ATmega88__) || defined(__AVR_ATmega168P__) || defined(__AVR_ATmega168__) || defined(__AVR_ATmega328P__) || defined(__AVR_ATmega328__)
|
||||
//video
|
||||
#if defined(ENABLE_FAST_OUTPUT)
|
||||
#define PORT_VID PORTD
|
||||
#define DDR_VID DDRD
|
||||
#define VID_PIN 7
|
||||
#else
|
||||
#define PORT_VID PORTB
|
||||
#define DDR_VID DDRB
|
||||
#define VID_PIN 0
|
||||
#endif
|
||||
//sync
|
||||
#define PORT_SYNC PORTB
|
||||
#define DDR_SYNC DDRB
|
||||
#define SYNC_PIN 1
|
||||
//sound
|
||||
#define PORT_SND PORTB
|
||||
#define DDR_SND DDRB
|
||||
#define SND_PIN 3
|
||||
|
||||
// Arduino DUE
|
||||
#elif defined (__AVR_AT90USB1286__)
|
||||
//video
|
||||
#define PORT_VID PORTF
|
||||
#define DDR_VID DDRF
|
||||
#define VID_PIN 7
|
||||
//sync
|
||||
#define PORT_SYNC PORTB
|
||||
#define DDR_SYNC DDRB
|
||||
#define SYNC_PIN 5
|
||||
//sound
|
||||
#define PORT_SND PORTB
|
||||
#define DDR_SND DDRB
|
||||
#define SND_PIN 4
|
||||
|
||||
// Arduino Leonardo
|
||||
#elif defined(__AVR_ATmega32U4__)
|
||||
// video arduino pin 8
|
||||
#define PORT_VID PORTB
|
||||
#define DDR_VID DDRB
|
||||
#define VID_PIN 4
|
||||
// sync arduino pin 9
|
||||
#define PORT_SYNC PORTB
|
||||
#define DDR_SYNC DDRB
|
||||
#define SYNC_PIN 5
|
||||
// sound arduino pin 11
|
||||
#define PORT_SND PORTB
|
||||
#define DDR_SND DDRB
|
||||
#define SND_PIN 7
|
||||
#define TCCR2A TCCR0A
|
||||
#define TCCR2B TCCR0B
|
||||
#define OCR2A OCR0A
|
||||
#define OCR2B OCR0B
|
||||
#define COM2A0 COM0A0
|
||||
#define COM2A1 COM0A1
|
||||
#define CS20 CS00
|
||||
#define WGM21 WGM01
|
||||
#endif
|
||||
|
||||
//automatic BST/BLD/ANDI macro definition
|
||||
#if VID_PIN == 0
|
||||
#define BLD_HWS "bld r16,0\n\t"
|
||||
#define BST_HWS "bst r16,0\n\t"
|
||||
#define ANDI_HWS "andi r16,0xFE\n"
|
||||
#elif VID_PIN == 1
|
||||
#define BLD_HWS "bld r16,1\n\t"
|
||||
#define BST_HWS "bst r16,1\n\t"
|
||||
#define ANDI_HWS "andi r16,0xFD\n"
|
||||
#elif VID_PIN == 2
|
||||
#define BLD_HWS "bld r16,2\n\t"
|
||||
#define BST_HWS "bst r16,2\n\t"
|
||||
#define ANDI_HWS "andi r16,0xFB\n"
|
||||
#elif VID_PIN == 3
|
||||
#define BLD_HWS "bld r16,3\n\t"
|
||||
#define BST_HWS "bst r16,3\n\t"
|
||||
#define ANDI_HWS "andi r16,0xF7\n"
|
||||
#elif VID_PIN == 4
|
||||
#define BLD_HWS "bld r16,4\n\t"
|
||||
#define BST_HWS "bst r16,4\n\t"
|
||||
#define ANDI_HWS "andi r16,0xEF\n"
|
||||
#elif VID_PIN == 5
|
||||
#define BLD_HWS "bld r16,5\n\t"
|
||||
#define BST_HWS "bst r16,5\n\t"
|
||||
#define ANDI_HWS "andi r16,0xDF\n"
|
||||
#elif VID_PIN == 6
|
||||
#define BLD_HWS "bld r16,6\n\t"
|
||||
#define BST_HWS "bst r16,6\n\t"
|
||||
#define ANDI_HWS "andi r16,0xBF\n"
|
||||
#elif VID_PIN == 7
|
||||
#define BLD_HWS "bld r16,7\n\t"
|
||||
#define BST_HWS "bst r16,7\n\t"
|
||||
#define ANDI_HWS "andi r16,0x7F\n"
|
||||
#endif
|
||||
#endif
|
|
@ -1,41 +0,0 @@
|
|||
/* This File contains the timing definitions for the TVout AVR composite video
|
||||
generation Library
|
||||
*/
|
||||
#ifndef VIDEO_TIMING_H
|
||||
#define VIDEO_TIMING_H
|
||||
|
||||
#define _CYCLES_PER_US (F_CPU / 1000000)
|
||||
|
||||
#define _TIME_HORZ_SYNC 4.7
|
||||
#define _TIME_VIRT_SYNC 58.85
|
||||
#define _TIME_ACTIVE 46
|
||||
#define _CYCLES_VIRT_SYNC ((_TIME_VIRT_SYNC * _CYCLES_PER_US) - 1)
|
||||
#define _CYCLES_HORZ_SYNC ((_TIME_HORZ_SYNC * _CYCLES_PER_US) - 1)
|
||||
|
||||
// Timing settings for NTSC
|
||||
#define _NTSC_TIME_SCANLINE 63.55
|
||||
#define _NTSC_TIME_OUTPUT_START 12
|
||||
|
||||
#define _NTSC_LINE_FRAME 262
|
||||
#define _NTSC_LINE_START_VSYNC 0
|
||||
#define _NTSC_LINE_STOP_VSYNC 3
|
||||
#define _NTSC_LINE_DISPLAY 216
|
||||
#define _NTSC_LINE_MID ((_NTSC_LINE_FRAME - _NTSC_LINE_DISPLAY) / 2 + _NTSC_LINE_DISPLAY / 2)
|
||||
|
||||
#define _NTSC_CYCLES_SCANLINE ((_NTSC_TIME_SCANLINE * _CYCLES_PER_US) - 1)
|
||||
#define _NTSC_CYCLES_OUTPUT_START ((_NTSC_TIME_OUTPUT_START * _CYCLES_PER_US) - 1)
|
||||
|
||||
// Timing settings for PAL
|
||||
#define _PAL_TIME_SCANLINE 64
|
||||
#define _PAL_TIME_OUTPUT_START 12.5
|
||||
|
||||
#define _PAL_LINE_FRAME 312
|
||||
#define _PAL_LINE_START_VSYNC 0
|
||||
#define _PAL_LINE_STOP_VSYNC 7
|
||||
#define _PAL_LINE_DISPLAY 260
|
||||
#define _PAL_LINE_MID ((_PAL_LINE_FRAME - _PAL_LINE_DISPLAY) / 2 + _PAL_LINE_DISPLAY / 2)
|
||||
|
||||
#define _PAL_CYCLES_SCANLINE ((_PAL_TIME_SCANLINE * _CYCLES_PER_US) - 1)
|
||||
#define _PAL_CYCLES_OUTPUT_START ((_PAL_TIME_OUTPUT_START * _CYCLES_PER_US) - 1)
|
||||
|
||||
#endif
|
|
@ -1,517 +0,0 @@
|
|||
/*
|
||||
Copyright (c) 2010 Myles Metzer
|
||||
Permission is hereby granted, free of charge, to any person
|
||||
obtaining a copy of this software and associated documentation
|
||||
files (the "Software"), to deal in the Software without
|
||||
restriction, including without limitation the rights to use,
|
||||
copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the
|
||||
Software is furnished to do so, subject to the following
|
||||
conditions:
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
|
||||
OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
|
||||
HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
|
||||
WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
|
||||
FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
|
||||
OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include "video_gen.h"
|
||||
#include "spec/asm_macros.h"
|
||||
|
||||
//#define REMOVE6C
|
||||
//#define REMOVE5C
|
||||
//#define REMOVE4C
|
||||
//#define REMOVE3C
|
||||
|
||||
int renderLine;
|
||||
TVout_vid display;
|
||||
void (*render_line)();
|
||||
void (*line_handler)();
|
||||
void (*hbi_hook)() = ∅
|
||||
void (*vbi_hook)() = ∅
|
||||
|
||||
// sound properties
|
||||
volatile long remainingToneVsyncs;
|
||||
|
||||
void empty() {
|
||||
|
||||
}
|
||||
// start timer
|
||||
void render_setup(uint8_t mode, uint8_t x, uint8_t y, uint8_t *scrnptr) {
|
||||
display.screen = scrnptr;
|
||||
display.hres = x;
|
||||
display.vres = y;
|
||||
display.frames = 0;
|
||||
|
||||
if (mode) {
|
||||
display.vscale_const = _PAL_LINE_DISPLAY / display.vres - 1;
|
||||
} else {
|
||||
display.vscale_const = _NTSC_LINE_DISPLAY / display.vres - 1;
|
||||
}
|
||||
display.vscale = display.vscale_const;
|
||||
|
||||
// selects the widest render method that fits in 46us
|
||||
// as of 9/16/10 rendermode 3 will not work for resolutions lower than
|
||||
// 192(display.hres lower than 24)
|
||||
unsigned char rmethod = (_TIME_ACTIVE*_CYCLES_PER_US) / (display.hres * 8);
|
||||
switch(rmethod) {
|
||||
case 6:
|
||||
render_line = &render_line6c;
|
||||
break;
|
||||
case 5:
|
||||
render_line = &render_line5c;
|
||||
break;
|
||||
case 4:
|
||||
render_line = &render_line4c;
|
||||
break;
|
||||
case 3:
|
||||
render_line = &render_line3c;
|
||||
break;
|
||||
default:
|
||||
if (rmethod > 6) {
|
||||
render_line = &render_line6c;
|
||||
} else {
|
||||
render_line = &render_line3c;
|
||||
}
|
||||
}
|
||||
|
||||
DDR_VID |= _BV(VID_PIN);
|
||||
DDR_SYNC |= _BV(SYNC_PIN);
|
||||
PORT_VID &= ~_BV(VID_PIN);
|
||||
PORT_SYNC |= _BV(SYNC_PIN);
|
||||
// for tone generation
|
||||
DDR_SND |= _BV(SND_PIN);
|
||||
|
||||
// inverted fast pwm mode on timer 1
|
||||
TCCR1A = _BV(COM1A1) | _BV(COM1A0) | _BV(WGM11);
|
||||
TCCR1B = _BV(WGM13) | _BV(WGM12) | _BV(CS10);
|
||||
|
||||
if (mode) {
|
||||
display.start_render = _PAL_LINE_MID - ((display.vres * (display.vscale_const + 1)) / 2);
|
||||
display.output_delay = _PAL_CYCLES_OUTPUT_START;
|
||||
display.vsync_end = _PAL_LINE_STOP_VSYNC;
|
||||
display.lines_frame = _PAL_LINE_FRAME;
|
||||
ICR1 = _PAL_CYCLES_SCANLINE;
|
||||
OCR1A = _CYCLES_HORZ_SYNC;
|
||||
} else {
|
||||
display.start_render = _NTSC_LINE_MID - ((display.vres * (display.vscale_const+1))/2) + 8;
|
||||
display.output_delay = _NTSC_CYCLES_OUTPUT_START;
|
||||
display.vsync_end = _NTSC_LINE_STOP_VSYNC;
|
||||
display.lines_frame = _NTSC_LINE_FRAME;
|
||||
ICR1 = _NTSC_CYCLES_SCANLINE;
|
||||
OCR1A = _CYCLES_HORZ_SYNC;
|
||||
}
|
||||
display.scanLine = display.lines_frame + 1;
|
||||
line_handler = &vsync_line;
|
||||
TIMSK1 = _BV(TOIE1);
|
||||
sei();
|
||||
}
|
||||
|
||||
// render a line
|
||||
ISR(TIMER1_OVF_vect) {
|
||||
hbi_hook();
|
||||
line_handler();
|
||||
}
|
||||
|
||||
// вызываем синхронизацию или прорисовку строки
|
||||
void blank_line() {
|
||||
if (display.scanLine == display.start_render) {
|
||||
renderLine = 0;
|
||||
display.vscale = display.vscale_const;
|
||||
line_handler = &active_line;
|
||||
} else if (display.scanLine == display.lines_frame) {
|
||||
line_handler = &vsync_line;
|
||||
vbi_hook();
|
||||
}
|
||||
display.scanLine++;
|
||||
}
|
||||
|
||||
|
||||
void active_line() {
|
||||
wait_until(display.output_delay);
|
||||
render_line();
|
||||
if (!display.vscale) {
|
||||
display.vscale = display.vscale_const;
|
||||
renderLine += display.hres;
|
||||
} else {
|
||||
display.vscale--;
|
||||
}
|
||||
if ((display.scanLine + 1) == (int)(display.start_render + (display.vres * (display.vscale_const + 1)))) {
|
||||
line_handler = &blank_line;
|
||||
}
|
||||
display.scanLine++;
|
||||
}
|
||||
|
||||
// генерируем синхроимпульс
|
||||
void vsync_line() {
|
||||
if (display.scanLine >= display.lines_frame) {
|
||||
OCR1A = _CYCLES_VIRT_SYNC;
|
||||
display.scanLine = 0;
|
||||
display.frames++;
|
||||
if (remainingToneVsyncs != 0) {
|
||||
if (remainingToneVsyncs > 0) {
|
||||
remainingToneVsyncs--;
|
||||
}
|
||||
} else {
|
||||
// stop the tone
|
||||
TCCR2B = 0;
|
||||
PORTB &= ~(_BV(SND_PIN));
|
||||
}
|
||||
} else if (display.scanLine == display.vsync_end) {
|
||||
OCR1A = _CYCLES_HORZ_SYNC;
|
||||
line_handler = &blank_line;
|
||||
}
|
||||
display.scanLine++;
|
||||
}
|
||||
|
||||
|
||||
static void inline wait_until(uint8_t time) {
|
||||
__asm__ __volatile__ (
|
||||
"subi %[time], 10\n"
|
||||
"sub %[time], %[tcnt1l]\n\t"
|
||||
"100:\n\t"
|
||||
"subi %[time], 3\n\t"
|
||||
"brcc 100b\n\t"
|
||||
"subi %[time], 0-3\n\t"
|
||||
"breq 101f\n\t"
|
||||
"dec %[time]\n\t"
|
||||
"breq 102f\n\t"
|
||||
"rjmp 102f\n"
|
||||
"101:\n\t"
|
||||
"nop\n"
|
||||
"102:\n"
|
||||
:
|
||||
: [time] "a" (time),
|
||||
[tcnt1l] "a" (TCNT1L)
|
||||
);
|
||||
}
|
||||
|
||||
void render_line6c() {
|
||||
#ifndef REMOVE6C
|
||||
__asm__ __volatile__ (
|
||||
"ADD r26,r28\n\t"
|
||||
"ADC r27,r29\n\t"
|
||||
// save PORTB
|
||||
"svprt %[port]\n\t"
|
||||
"rjmp enter6\n"
|
||||
"loop6:\n\t"
|
||||
// 8
|
||||
"bst __tmp_reg__,0\n\t"
|
||||
"o1bs %[port]\n"
|
||||
"enter6:\n\t"
|
||||
// 1
|
||||
"LD __tmp_reg__,X+\n\t"
|
||||
"delay1\n\t"
|
||||
"bst __tmp_reg__,7\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// 2
|
||||
"delay3\n\t"
|
||||
"bst __tmp_reg__,6\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// 3
|
||||
"delay3\n\t"
|
||||
"bst __tmp_reg__,5\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// 4
|
||||
"delay3\n\t"
|
||||
"bst __tmp_reg__,4\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// 5
|
||||
"delay3\n\t"
|
||||
"bst __tmp_reg__,3\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// 6
|
||||
"delay3\n\t"
|
||||
"bst __tmp_reg__,2\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// 7
|
||||
"delay3\n\t"
|
||||
"bst __tmp_reg__,1\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
"dec %[hres]\n\t"
|
||||
// go too loopsix
|
||||
"brne loop6\n\t"
|
||||
"delay2\n\t"
|
||||
// 8
|
||||
"bst __tmp_reg__,0\n\t"
|
||||
"o1bs %[port]\n"
|
||||
"svprt %[port]\n\t"
|
||||
BST_HWS
|
||||
"o1bs %[port]\n\t"
|
||||
:
|
||||
: [port] "i" (_SFR_IO_ADDR(PORT_VID)),
|
||||
"x" (display.screen),
|
||||
"y" (renderLine),
|
||||
[hres] "d" (display.hres)
|
||||
: "r16" // try to remove this clobber later...
|
||||
);
|
||||
#endif
|
||||
}
|
||||
|
||||
void render_line5c() {
|
||||
#ifndef REMOVE5C
|
||||
__asm__ __volatile__ (
|
||||
"ADD r26,r28\n\t"
|
||||
"ADC r27,r29\n\t"
|
||||
// save PORTB
|
||||
"svprt %[port]\n\t"
|
||||
"rjmp enter5\n"
|
||||
"loop5:\n\t"
|
||||
// 8
|
||||
"bst __tmp_reg__,0\n\t"
|
||||
"o1bs %[port]\n"
|
||||
"enter5:\n\t"
|
||||
// 1
|
||||
"LD __tmp_reg__,X+\n\t"
|
||||
"bst __tmp_reg__,7\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// 2
|
||||
"delay2\n\t"
|
||||
"bst __tmp_reg__,6\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// 3
|
||||
"delay2\n\t"
|
||||
"bst __tmp_reg__,5\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// 4
|
||||
"delay2\n\t"
|
||||
"bst __tmp_reg__,4\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// 5
|
||||
"delay2\n\t"
|
||||
"bst __tmp_reg__,3\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// 6
|
||||
"delay2\n\t"
|
||||
"bst __tmp_reg__,2\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// 7
|
||||
"delay1\n\t"
|
||||
"dec %[hres]\n\t"
|
||||
"bst __tmp_reg__,1\n\t"
|
||||
"o1bs %[port]\n\t"
|
||||
// go too loop5
|
||||
"brne loop5\n\t"
|
||||
"delay1\n\t"
|
||||
// 8
|
||||
"bst __tmp_reg__,0\n\t"
|
||||
"o1bs %[port]\n"
|
||||
"svprt %[port]\n\t"
|
||||
BST_HWS
|
||||
"o1bs %[port]\n\t"
|
||||
:
|
||||
: [port] "i" (_SFR_IO_ADDR(PORT_VID)),
|
||||
"x" (display.screen),
|
||||
"y" (renderLine),
|
||||
[hres] "d" (display.hres)
|
||||
: "r16" // try to remove this clobber later...
|
||||
);
|
||||
#endif
|
||||
}
|
||||
|
||||
void render_line4c() {
|
||||
#ifndef REMOVE4C
|
||||
__asm__ __volatile__ (
|
||||
"ADD r26,r28\n\t"
|
||||
"ADC r27,r29\n\t"
|
||||
"rjmp enter4\n"
|
||||
"loop4:\n\t"
|
||||
// 8
|
||||
"lsl __tmp_reg__\n\t"
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
"enter4:\n\t"
|
||||
// 1
|
||||
"LD __tmp_reg__,X+\n\t"
|
||||
"delay1\n\t"
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
// 2
|
||||
"delay2\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
// 3
|
||||
"delay2\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
// 4
|
||||
"delay2\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
"delay2\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
// 6
|
||||
"delay2\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
// 7
|
||||
"delay1\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
"dec %[hres]\n\t"
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
// go too loop4
|
||||
"brne loop4\n\t"
|
||||
// 8
|
||||
"delay1\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
"delay3\n\t"
|
||||
"cbi %[port],7\n\t"
|
||||
:
|
||||
: [port] "i" (_SFR_IO_ADDR(PORT_VID)),
|
||||
"x" (display.screen),
|
||||
"y" (renderLine),
|
||||
[hres] "d" (display.hres)
|
||||
: "r16" // try to remove this clobber later...
|
||||
);
|
||||
#endif
|
||||
}
|
||||
|
||||
// only 16mhz right now!!!
|
||||
void render_line3c() {
|
||||
#ifndef REMOVE3C
|
||||
__asm__ __volatile__ (
|
||||
".macro byteshift\n\t"
|
||||
"LD __tmp_reg__,X+\n\t"
|
||||
// 0
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
"nop\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
// 1
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
"nop\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
// 2
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
"nop\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
// 3
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
"nop\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
// 4
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
"nop\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
// 5
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
"nop\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
// 6
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
"nop\n\t"
|
||||
"lsl __tmp_reg__\n\t"
|
||||
// 7
|
||||
"out %[port],__tmp_reg__\n\t"
|
||||
".endm\n\t"
|
||||
"ADD r26,r28\n\t"
|
||||
"ADC r27,r29\n\t"
|
||||
// 615
|
||||
"cpi %[hres],30\n\t"
|
||||
"breq skip0\n\t"
|
||||
"cpi %[hres],29\n\t"
|
||||
"breq jumpto1\n\t"
|
||||
"cpi %[hres],28\n\t"
|
||||
"breq jumpto2\n\t"
|
||||
"cpi %[hres],27\n\t"
|
||||
"breq jumpto3\n\t"
|
||||
"cpi %[hres],26\n\t"
|
||||
"breq jumpto4\n\t"
|
||||
"cpi %[hres],25\n\t"
|
||||
"breq jumpto5\n\t"
|
||||
"cpi %[hres],24\n\t"
|
||||
"breq jumpto6\n\t"
|
||||
"jumpto1:\n\t"
|
||||
"rjmp skip1\n\t"
|
||||
"jumpto2:\n\t"
|
||||
"rjmp skip2\n\t"
|
||||
"jumpto3:\n\t"
|
||||
"rjmp skip3\n\t"
|
||||
"jumpto4:\n\t"
|
||||
"rjmp skip4\n\t"
|
||||
"jumpto5:\n\t"
|
||||
"rjmp skip5\n\t"
|
||||
"jumpto6:\n\t"
|
||||
"rjmp skip6\n\t"
|
||||
"skip0:\n\t"
|
||||
//1 643
|
||||
"byteshift\n\t"
|
||||
"skip1:\n\t"
|
||||
// 2
|
||||
"byteshift\n\t"
|
||||
"skip2:\n\t"
|
||||
// 3
|
||||
"byteshift\n\t"
|
||||
"skip3:\n\t"
|
||||
// 4
|
||||
"byteshift\n\t"
|
||||
"skip4:\n\t"
|
||||
// 5
|
||||
"byteshift\n\t"
|
||||
"skip5:\n\t"
|
||||
// 6
|
||||
"byteshift\n\t"
|
||||
"skip6:\n\t"
|
||||
// 7
|
||||
"byteshift\n\t"
|
||||
// 8
|
||||
"byteshift\n\t"
|
||||
// 9
|
||||
"byteshift\n\t"
|
||||
// 10
|
||||
"byteshift\n\t"
|
||||
// 11
|
||||
"byteshift\n\t"
|
||||
// 12
|
||||
"byteshift\n\t"
|
||||
// 13
|
||||
"byteshift\n\t"
|
||||
// 14
|
||||
"byteshift\n\t"
|
||||
// 15
|
||||
"byteshift\n\t"
|
||||
// 16
|
||||
"byteshift\n\t"
|
||||
// 17
|
||||
"byteshift\n\t"
|
||||
// 18
|
||||
"byteshift\n\t"
|
||||
// 19
|
||||
"byteshift\n\t"
|
||||
// 20
|
||||
"byteshift\n\t"
|
||||
// 21
|
||||
"byteshift\n\t"
|
||||
// 22
|
||||
"byteshift\n\t"
|
||||
// 23
|
||||
"byteshift\n\t"
|
||||
// 24
|
||||
"byteshift\n\t"
|
||||
// 25
|
||||
"byteshift\n\t"
|
||||
// 26
|
||||
"byteshift\n\t"
|
||||
// 27
|
||||
"byteshift\n\t"
|
||||
// 28
|
||||
"byteshift\n\t"
|
||||
// 29
|
||||
"byteshift\n\t"
|
||||
// 30
|
||||
"byteshift\n\t"
|
||||
"delay2\n\t"
|
||||
"cbi %[port],7\n\t"
|
||||
:
|
||||
: [port] "i" (_SFR_IO_ADDR(PORT_VID)),
|
||||
"x" (display.screen),
|
||||
"y" (renderLine),
|
||||
[hres] "d" (display.hres)
|
||||
: "r16" // try to remove this clobber later...
|
||||
);
|
||||
#endif
|
||||
}
|
|
@ -1,71 +0,0 @@
|
|||
/*
|
||||
Copyright (c) 2010 Myles Metzer
|
||||
Permission is hereby granted, free of charge, to any person
|
||||
obtaining a copy of this software and associated documentation
|
||||
files (the "Software"), to deal in the Software without
|
||||
restriction, including without limitation the rights to use,
|
||||
copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the
|
||||
Software is furnished to do so, subject to the following
|
||||
conditions:
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
|
||||
OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
|
||||
HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
|
||||
WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
|
||||
FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
|
||||
OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#ifndef VIDEO_GEN_H
|
||||
#define VIDEO_GEN_H
|
||||
|
||||
#include <avr/interrupt.h>
|
||||
#include <avr/io.h>
|
||||
#include "spec/video_properties.h"
|
||||
#include "spec/hardware_setup.h"
|
||||
|
||||
typedef struct {
|
||||
volatile int scanLine;
|
||||
volatile unsigned long frames;
|
||||
unsigned char start_render;
|
||||
// remove me
|
||||
int lines_frame;
|
||||
uint8_t vres;
|
||||
uint8_t hres;
|
||||
// remove me
|
||||
uint8_t output_delay;
|
||||
// combine me with status switch
|
||||
char vscale_const;
|
||||
// combine me too.
|
||||
char vscale;
|
||||
// remove me
|
||||
char vsync_end;
|
||||
uint8_t* screen;
|
||||
} TVout_vid;
|
||||
|
||||
extern TVout_vid display;
|
||||
|
||||
extern void (*hbi_hook)();
|
||||
extern void (*vbi_hook)();
|
||||
|
||||
void render_setup(uint8_t mode, uint8_t x, uint8_t y, uint8_t *scrnptr);
|
||||
|
||||
void blank_line();
|
||||
void active_line();
|
||||
void vsync_line();
|
||||
void empty();
|
||||
|
||||
// tone generation properties
|
||||
extern volatile long remainingToneVsyncs;
|
||||
|
||||
// 6cycles functions
|
||||
void render_line6c();
|
||||
void render_line5c();
|
||||
void render_line4c();
|
||||
void render_line3c();
|
||||
static void inline wait_until(uint8_t time);
|
||||
#endif
|
Before Width: | Height: | Size: 2.3 KiB After Width: | Height: | Size: 2.3 KiB |
Before Width: | Height: | Size: 2.4 KiB After Width: | Height: | Size: 2.4 KiB |
Before Width: | Height: | Size: 2.4 KiB After Width: | Height: | Size: 2.4 KiB |
Before Width: | Height: | Size: 2.3 KiB After Width: | Height: | Size: 2.3 KiB |
Before Width: | Height: | Size: 942 B After Width: | Height: | Size: 942 B |
Before Width: | Height: | Size: 955 B After Width: | Height: | Size: 955 B |
Before Width: | Height: | Size: 2.3 KiB After Width: | Height: | Size: 2.3 KiB |
Before Width: | Height: | Size: 2.2 KiB After Width: | Height: | Size: 2.2 KiB |
Before Width: | Height: | Size: 6.0 KiB After Width: | Height: | Size: 6.0 KiB |
Before Width: | Height: | Size: 6.2 KiB After Width: | Height: | Size: 6.2 KiB |
Before Width: | Height: | Size: 6.6 KiB After Width: | Height: | Size: 6.6 KiB |
Before Width: | Height: | Size: 6.4 KiB After Width: | Height: | Size: 6.4 KiB |
49
README.md
|
@ -1,27 +1,31 @@
|
|||
# SmartyKit Apple I - apple1
|
||||
SmartyKit Apple I replica drivers and software (http://www.smartykit.io/).
|
||||
# SmartyKit One Apple-1 compatible computer repo structure
|
||||
|
||||
SmartyKit One Apple-1 compatible computer's drivers and software (http://www.smartykit.io/):
|
||||
* **Controllers drivers** – drivers for all used controllers (Video, Keyboard & Hi-Res Video)
|
||||
- *Keyboard driver* uses Arduino PS2KeyAdvanced library.
|
||||
- *Video driver* uses Arduino custom 2.8" TFT screen driver (based on Adafruit driver) and TV Terminal library.
|
||||
- *Hi-Res Video driver* uses library written by Grant Searle and Dave Curran
|
||||
* **Garage ASCII art** – beautiful Steve Job's garage ASCII art by Adel Faure
|
||||
|
||||
* **ROM** – ROM chip firmwarwe source code (6502 assembler) & development configs (Makefile to build your own version of ROM using `ca65` 6502 assembler & config files to run the code in POM1 emulator)
|
||||
|
||||
* **POM1 software emulator** – Apple-1 software emulator by Verhille Arnaud to test SmartyKit ROM code before burning firmare
|
||||
|
||||

|
||||
|
||||
All needed libraries included in repository in /Arduino/libraries
|
||||
# Highlights
|
||||
|
||||
## Keyboard Driver
|
||||
Keyboard driver uses Arduino PS2KeyAdvanced library.
|
||||
## SmartyKit One's loading splash screen ASCII art
|
||||
Steve Job's garage ASCII art by Adel Faure
|
||||

|
||||
|
||||
## Video Driver
|
||||
Video driver uses Arduino custom 2.8" TFT screen driver (based on Adafruit driver) and TV Terminal library.
|
||||
|
||||
## Software Emulator - POM 1
|
||||
POM 1 cross-platform emulator by Verhille Arnaud to test SmartyKit 1 ROM with software.
|
||||
## SmartyKit One's ROM to run on POM1 Software Emulator
|
||||
POM 1 cross-platform emulator by Verhille Arnaud to test SmartyKit One ROM with software.
|
||||
|
||||
[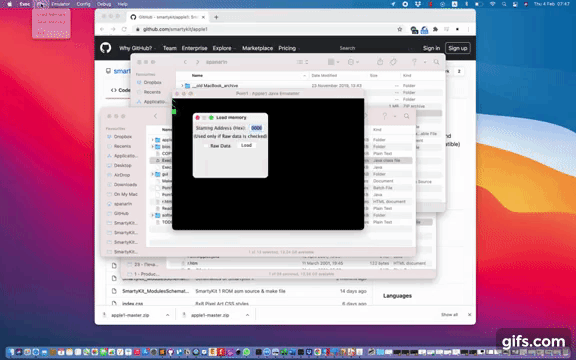](https://youtu.be/rniZDdS6toI)
|
||||
|
||||
## SmartyKit 1 Memory Map – ROM and RAM
|
||||
|
||||

|
||||
|
||||
## ROM Development – SmartyKit 1 ROM asm source and make file
|
||||
Easy-to-use development tool to write your own software for SmartyKLit 1 (you need just run make in your Terminal) and learn how ROM is organized.
|
||||
## SmartyKit One's ROM development console
|
||||
Easy-to-use development tool to write your own software for SmartyKLit One (you need just run make in your Terminal) and learn how ROM is organized.
|
||||
|
||||
Here is an example:
|
||||
```bash
|
||||
|
@ -34,21 +38,14 @@ ld65 -o SmartyKit1_ROM_symon.bin SmartyKit1_ROM.o -C symon.cfg
|
|||
SERGEYs-MacBook:ROM_development spanarin$
|
||||
```
|
||||
|
||||
## SmartyKit 8x8 Pixel Art online tool for program at $FC00 in ROM (FC00R command)
|
||||
You could draw your own pixel art using our online tool (https://smartykit.io/instructions/pixelart), get the code for this image and load this code it to SmartyKit (or emulator) memory using command:
|
||||
## SmartyKit One's 8x8 Pixel Art online tool for program at $FC00 in ROM (FC00R command)
|
||||
You could draw your own pixel art using our online tool (https://smartykit.github.io/pixelart-project/), get the code for this image and load this code it to SmartyKit (or emulator) memory using command:
|
||||
```
|
||||
1111: 3C 42 A5 81 A5 99 42 3C (Return)
|
||||
FC00R (Return)
|
||||
```
|
||||
|
||||
Here is an example of how it works in emulator:
|
||||
|
||||
[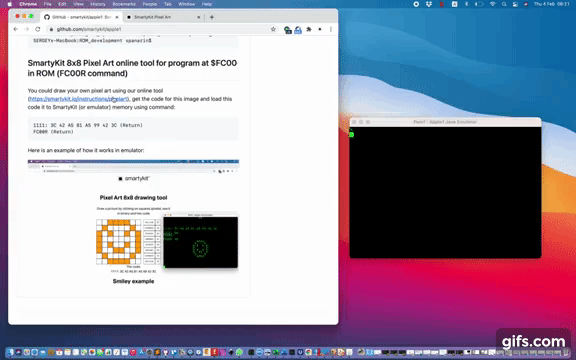](https://youtu.be/1KdQEKLvOHM)
|
||||
|
||||
And a screenshot:
|
||||
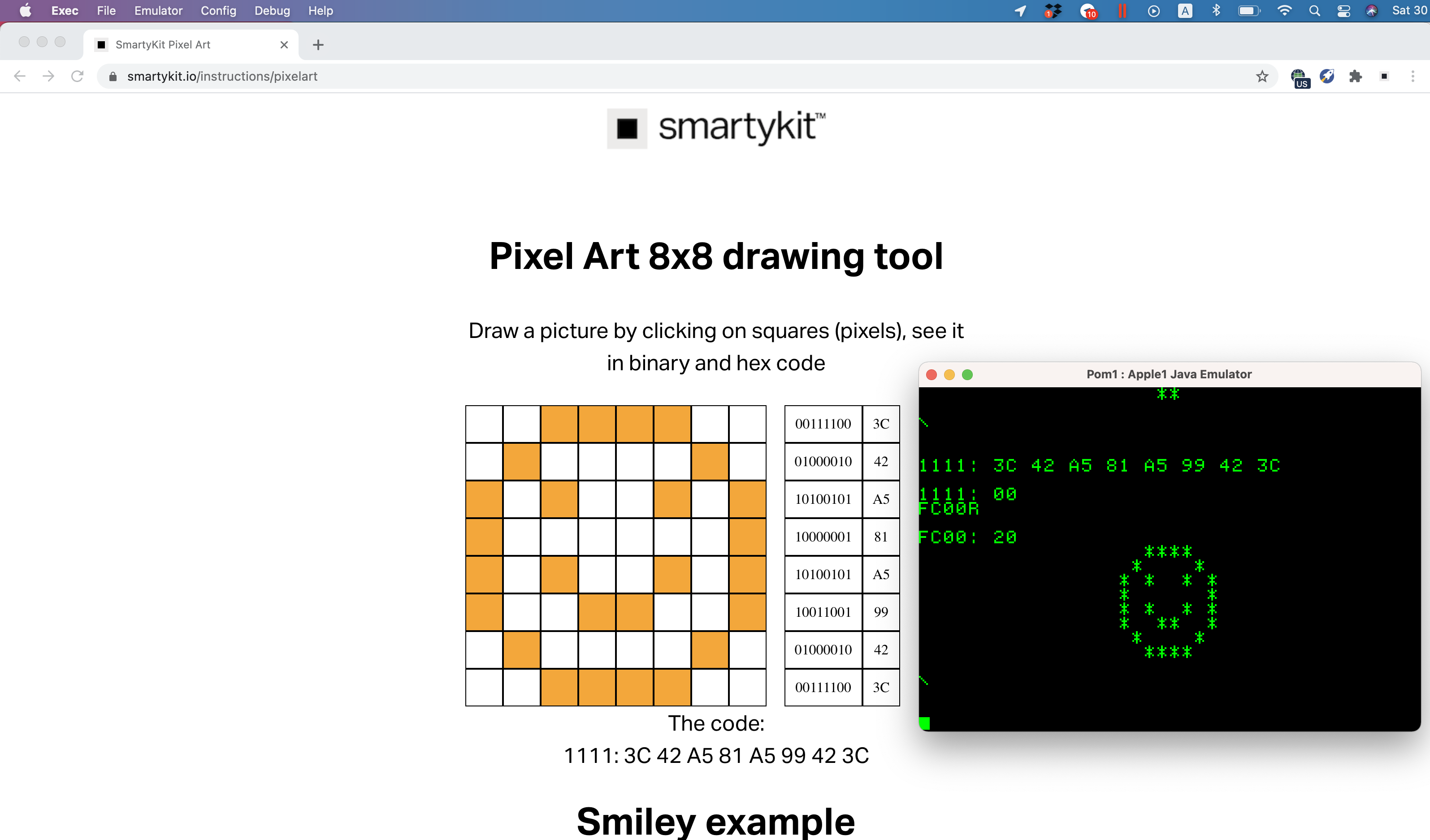
|
||||
|
||||
## SmartyKit plates for breadboards
|
||||
## SmartyKit One's plates for breadboards
|
||||
|
||||
PCB version (black) and Wooden version (file for wood laser cutter: [download](https://github.com/smartykit/apple1/blob/01ea2ec54a94a7a31ee613f007602f0c08cb13ae/SmartyKit-Plate-for-breadboards(laser-cut).cdr?raw=true))
|
||||
|
||||
|
|
Before Width: | Height: | Size: 95 KiB After Width: | Height: | Size: 95 KiB |